hello is me again.
i am trying to add rotation to my graphics engine but it is not going well. when the mesh rotates it becomes distorted, my first thought was that the problem was my line drawing implementation but after changing it the problem still occurred. what can i do the solve this issue.
--Line drawing code--
static void DrawLine(i32 x0, i32 y0, i32 x1, i32 y1, u32 Color)
{int x, y, dx, dy, px, py, dx1, dy1, xe, ye;
dx = x1 - x0; dy = y1 - y0;
if(dx==0) //Line Vertical
{
if(y1 < y0)
{
y0 = y0 + y1;
y1 = y0 - y1;
y0 = y0 - y1;
}
for(y = y0; y <= y1; y++) plotPixel(x0, y, Color);
}
if(dy==0)
{
if(x1 < x0)
{
x0 = x0 + x1;
x1 = x0 - x1;
x0 = x0 - x1;
}
for(x = x0; x <= x1; x++) plotPixel(x, y0, Color);
}
dx1 = abs(dx); dy1 = abs(dy);
px = 2 * dy1 - dx1; py = 2 * dx1 - dy1;
if(dx1 >= dy1)
{
if(dx >= 0)
{
x = x0; y = y0; xe = x1;
}else
{
x = x1; y = y1; xe = x0;
}
plotPixel(x,y,Color);
for (int i = 0; x < xe; i++)
{
x = x + 1;
if(px <= 0)
{
px = px + 2 * dy1;
}else
{
if((dx < 0 && dy < 0) || (dx > 0 && dy > 0))
{
y = y + 1;
}else
{
y = y - 1;
}
px = px + 2 * (dy1 - dx1);
plotPixel(x,y,Color);
}
}
}else
{
if(dy >= 0)
{
x = x0; y = y0; ye = y1;
}else
{
x = x1; y = y1; ye = y0;
}
plotPixel(x,y,Color);
for (int i = 0; y < ye; i++)
{
y = y + 1;
if(py <= 0)
{
py = py + 2 * dx1;
}else
{
if((dx < 0 && dy < 0) || (dx > 0 && dy > 0))
{
x = x + 1;
}else
{
x = x - 1;
}
py = py + 2 * (dy1 - dx1);
plotPixel(x,y,Color);
}
}
}
}
--Rotation & transform--
//vecShapes[0].angle = vecShapes[0].angle + 0.1 * dt;
for (int i = 0; i < 4; i++)
{
vecShapes[0].p_v[i] = (vec2D)
{
(vecShapes[0].o_v[i].x * cosf(vecShapes[0].angle)) - (vecShapes[0].o_v[i].y * sinf(vecShapes[0].angle)) + vecShapes[0].pos.x,
(vecShapes[0].o_v[i].x * sinf(vecShapes[0].angle)) + (vecShapes[0].o_v[i].y * cosf(vecShapes[0].angle)) + vecShapes[0].pos.y
};
}
--Drawing--
for (int i = 0; i < 4; i++)
{
DrawLine(vecShapes[0].p_v[i].x, vecShapes[0].p_v[i].y, vecShapes[0].p_v[(i+1)%4].x,vecShapes[0].p_v[(i+1)%4].y,0xfffffff);
}
--Without rotation--
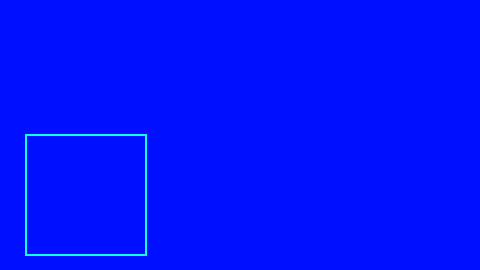
--With rotation--
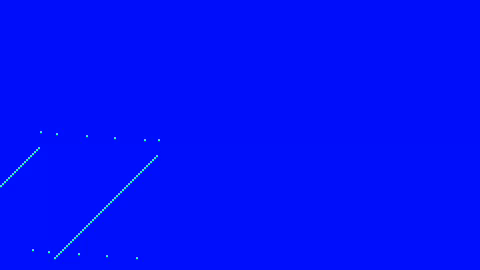