I am trying to fit an ellipse to some points along an orbit. The full code is at: https://github.com/sjhalayka/ellipse_fitting
As you can see, it sort of works, but not quite, especially where there are a lot of input points:
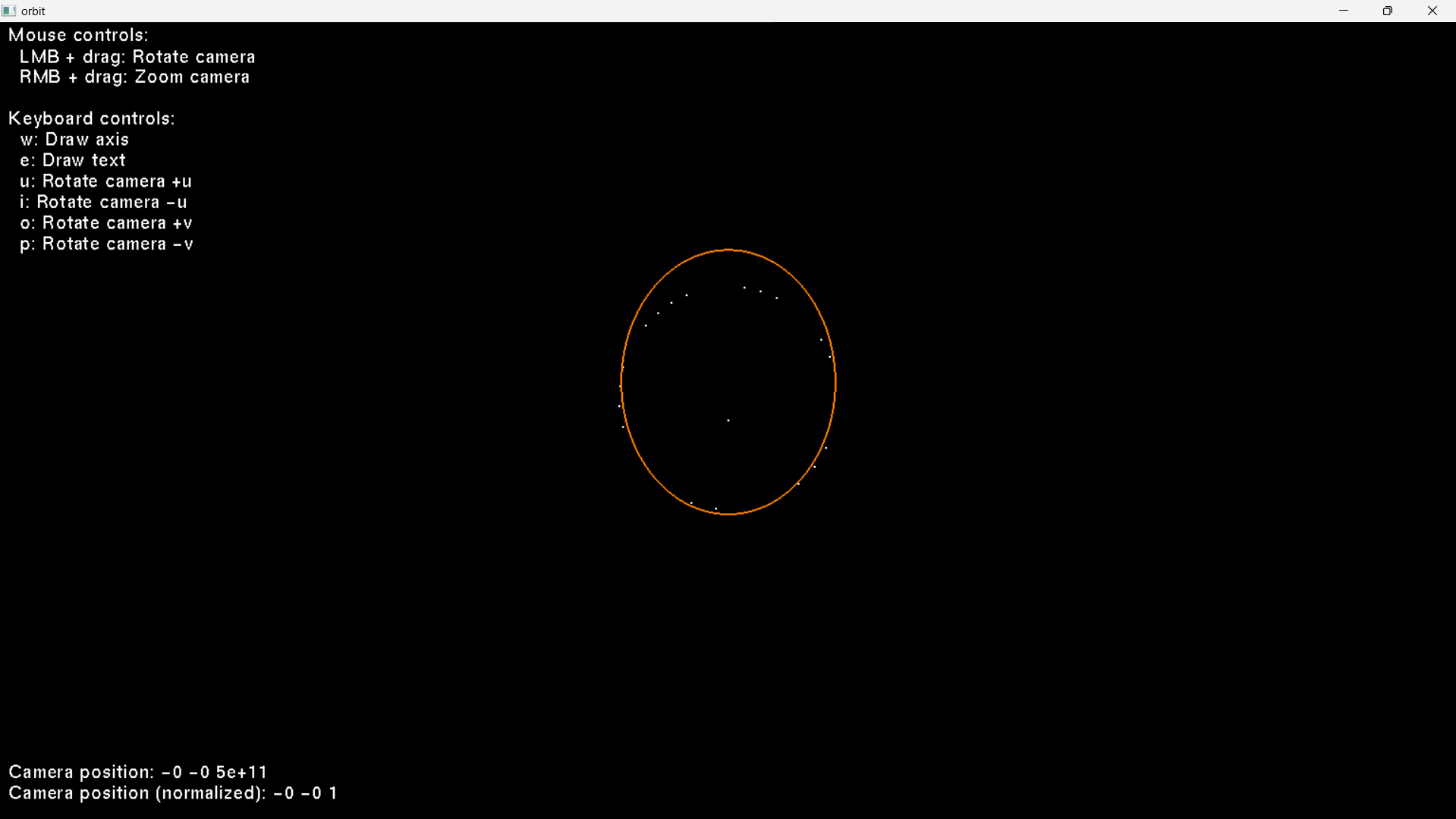
Here is the relevant code:
void idle_func(void)
{
static size_t frame_count = 0;
frame_count++;
const double dt = 10000; // 10000 seconds == 2.77777 hours
proceed_symplectic4(mercury_pos, mercury_vel, grav_constant, dt);
positions.push_back(mercury_pos);
static bool calculated_ellipse = false;
if (calculated_ellipse == false && frame_count % 123 == 0)
ellipse_positions.push_back(positions[positions.size() - 1]);
if (false == calculated_ellipse && ellipse_positions.size() == 20)
{
calculated_ellipse = true;
double largest_distance = 0;
for (size_t i = 0; i < ellipse_positions.size(); i++)
if (ellipse_positions[i].length() > largest_distance)
largest_distance = ellipse_positions[i].length();
global_ep.centerX = 0;
global_ep.centerY = 0;
global_ep.semiMajor = 10 * largest_distance;
global_ep.semiMinor = 10 * largest_distance;
double global_total_error = DBL_MAX;
for (size_t i = 1; i < 10000; i++)
{
EllipseParameters local_ep;
local_ep.centerX = global_ep.centerX;
local_ep.centerY = global_ep.centerY;
if (i % 2 == 0)
{
local_ep.semiMajor = global_ep.semiMajor;
local_ep.semiMinor = global_ep.semiMinor * 0.9;
}
else
{
local_ep.semiMajor = global_ep.semiMajor * 0.9;
local_ep.semiMinor = global_ep.semiMinor;
}
double local_total_error = 0;
for (size_t j = 0; j < ellipse_positions.size(); j++)
local_total_error += abs((ellipse_positions[j].x * ellipse_positions[j].x / (local_ep.semiMinor * local_ep.semiMinor)) + (ellipse_positions[j].y * ellipse_positions[j].y / (local_ep.semiMajor * local_ep.semiMajor)) - 1.0);
if (local_total_error < global_total_error)
{
global_ep.centerX = 0;
global_ep.centerY = 0.5*calculateFoci(local_ep).focus1X;
global_ep.semiMajor = local_ep.semiMajor;
global_ep.semiMinor = local_ep.semiMinor;
global_total_error = local_total_error;
}
}
}
glutPostRedisplay();
}
Any glaring errors in logic that I'm missing?