To simplify the question, I'm boiling down one of the mechanics of my game into two hexagons. The mechanic comes down to rotating the black hexagon to align with the red hexagon. The black hexagon will encounter red hexagons that are oriented around an arbitrary angle. The black hexagon will rotate smoothly to match the orientation of the next selected red hexagon. The red hexagons might not be perfect hexagons. That is, they might be slightly deformed and the angles of the corners might be bigger or smaller than 60 degrees.
The alignment portion of the rotation (getting all vertices to align with other vertices) is straightforward:
- Choose a reference vector (e.g. Vector2.UnitY)
- Determine the topmost vertex and calculate the angle between it and the reference vector
- Apply rotation with the given angle
The problem is that eventually, the orientation will have a full 60/-60 rotation in it. The reason is simple, depending on which hexagon selected next, the new “topmost” vertex might now be on the other side (e.g. shift from +x to -x).
At first, I figured that this is just a matter of selecting the topmost vertex with the smallest angle by comparing the absolutely value of the topmost and second topmost angles. But this makes no difference, eventually, the 60/-60 degree rotation will still be applied, because either side will have a new topmost vertex.
I tried a number of approaches including constraining the maximum orientation to 30 degrees (and then flipping to the other angle). But with every approach I try, at some point, I once again hit the 60 degree rotation, either in cases where the orientation reaching a flat top, or the angles are being exchanged.
Am I hitting some sort of a mathematic constraint and/or this simply not possible? Is there no way to orient one hexagon with another with an arbitrary angle without eventually doing a full 60-degree rotation?
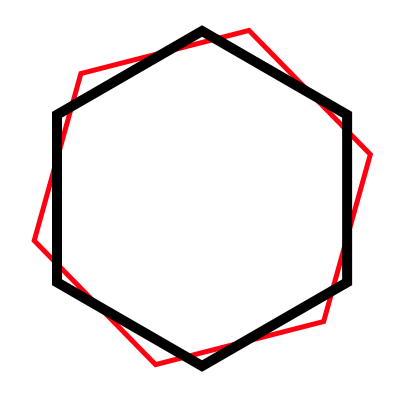