sorry you posted this code already, I am going to use it.
poker game
I used his code and have made some progress, here is my latest screen shot
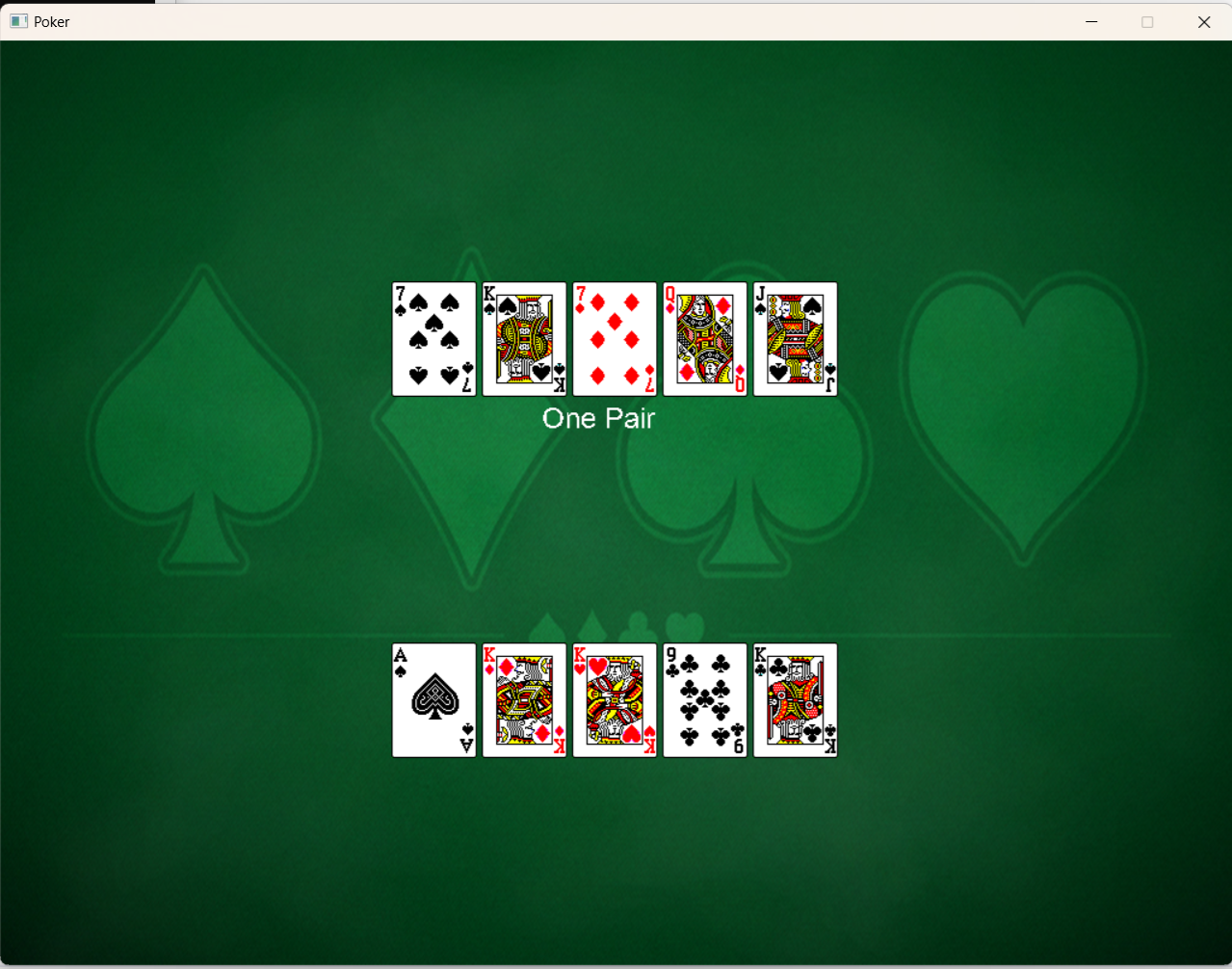
here is my latest version, however I have to do is straights and flush's I will work on this problem.
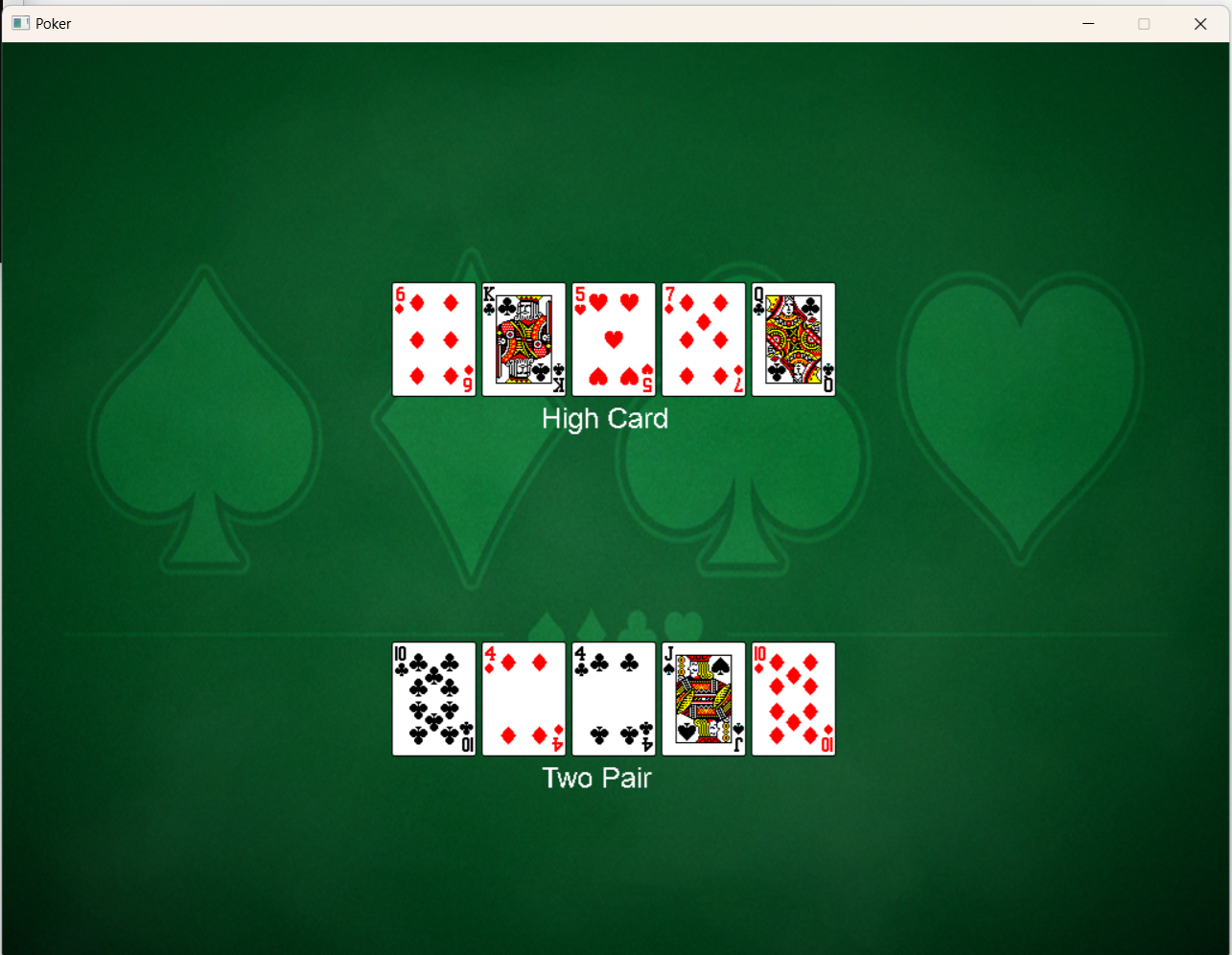
We showed that here.
http://gamedev.net/forums/topic/717647-poker-game/5467391/?page=3
After you realize that, the next step will be sorting and finalizing some logic.
Dev careful. Pixel on board.
🙂good to hear.
If you're ready to talk about sorting, we're going to have to be a little inventive.
The way we're set up now, our deck holds integers from 1 to 52.
We use std::shuffle to mix up the deck. (Nice and easy)
We count the modulo converted values to get one-pair, two-pair, three-of-kind, full-house or four-of-kind. (Bee's knees)
We use conditional statements on deck ranges to find flush. (Who'd a thunk it)
For the final step, we can finish in two different ways.
One way is, find the lowest card value and do messy loops and if statements to determine if the hand is sequential. (might actually be the way to go)
Or we can use std::sort to put in ascending order. https://www.digitalocean.com/community/tutorials/sort-in-c-plus-plus (easier read than msdn)
Either way, we should not be using the deck values (1 to 52) for the sort.
Instead use the modulo converted values. (0 to 12 except 0 should be 13 (remember why?))
The problem is, after a sort we lose the connection to the deck representation (1 to 52) using the standard template library route.
That's your clue. There is still work to be done.
Can't wait to see what you come up with.
my flush eval code for comparison
#include <bitset>
std::bitset<4> suit_bitset;
bool flush_found = false;
for (int i = 0; i < 5; i++)
{
if (hand->cards[i] < 14) suit_bitset.set(0); // clubs
if (hand->cards[i] > 13 && hand->cards[i] < 27) suit_bitset.set(1); // diamonds
if (hand->cards[i] > 26 && hand->cards[i] < 40) suit_bitset.set(2); // spades
if (hand->cards[i] > 39) suit_bitset.set(3); // hearts
}
if (suit_bitset.count() == 1) flush_found = true;
edit; Realized a way to keep the deck representation during sorting through lambda argument to std::sort
working snipit of my straight eval portion
std::sort(hand->cards, hand->cards + 5,
[](const int& first, const int& second) -> bool
{ // keeping connection to deck representation
int a = first % 13;
int b = second % 13;
return a < b;
}
);
Dev careful. Pixel on board.