After spending time with built-in vehicle component in Unreal Engine (WheelHandler) and Unity3D (WheelCollider), I realized that both of them don't have proper suspension movement. The wheels only go up and down on a vertical axis, like the spring-damper is connected right on top of the wheel. But in reality, it should move on an arc, and affect the camber, based on the type of suspension (leafspring, double wishbone, strout)
In my suspension implementation, I have a WheelHub component, that stays in place within the car body, acting as a suspension-wheel system. I only use one raycast model just like how WheelHandler and WheelCollider works. The WheelHub acts as the frame of reference, then other stuffs like steering angle, camber, suspension compression, are integrated in to a virtual wheel position and rotation, using Vector3 and Quaternion maths. Then the resulting rotation and position is applied to the actual mesh. Sort of like:
public void GetWheelPose(out Quaternion rotation, out Vector3 position)
{
// Maths here. Use wheelhub as reference frame since it doesn't move
// For example, steering
Quaternion upRotation = Quaternion.AngleAxis(wheelUpVector, steeringAngle);
rotation = wheelhub.rotation * upRotation;
// wheel position based on suspension compression
position = wheelhub.position + wheelhub.up * suspensionCompression;
}
Then the wheel mesh receives the about result to set its position and rotation (world coordinate)
GetWheelPose(out wheelMesh.rotation, out wheelMesh.position);
So my questions are:
1- When the wheel moves along an arc, it also changes its sideway position a bit, does the tire on the wheel experience slip/scrubbing and wear out over time? Assuming the tire is very sticky and will not move/slide sideway, when the wheel is at a point in the arc, how would the car body react to the force the tire is generating to hold it it place? Well, is that suspension movement correct at all in real life?
2 - What would be the Vector3 and Quaternion maths to calculate the position and rotation of the wheel based on the suspension travel? I don't want to use methods in Transform like Transform.RotateAround(), and only wants to use Quaternion and Vector3. Currently I need a rotation around a virtual axis, like the image below, then how can I do that using Quaternion math?
Thank you very much for your help. I'm sorry if I'm being hard to understand. English is not my first language, but I will try my best to explain myself.
Images taken from Unreal Documentation. They're not the mose accurate, but conveys the idea.
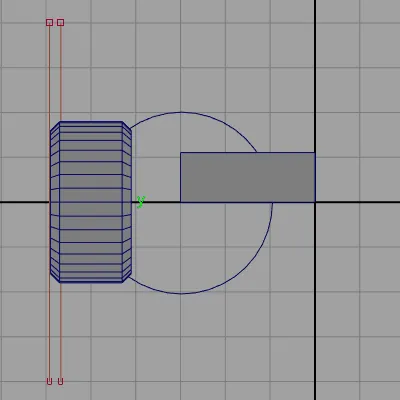
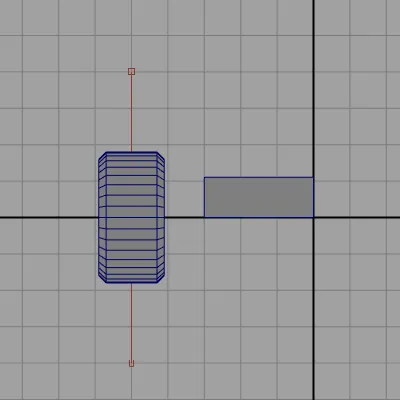