Edit: Basically, I'm looking to get the roll angle from a forward (a vector tangent to the surface of a sphere) and up vector. The yaw and pitch are gotten using the up vector.
I have some game pieces on a sphere, and I move them along pseudorandomly-generated geodesics (great circles). The only problem is that the pieces do not face forward, in the direction of its tangent vector. I tried many different things, but I cannot figure it out. Note that the fractal is aligned along the z axis.
The whole code is at: https://github.com/sjhalayka/opengl4_stlview_shadow_map
The pertinent code is:
void set_transform(void)
{
vec3 temp_dir = normalize(geodesic_dir);
float yaw = 0.0f;
if (fabsf(temp_dir.x) < 0.00001 && fabsf(temp_dir.z) < 0.00001)
yaw = 0.0f;
else
yaw = atan2f(temp_dir.x, temp_dir.z);
float pitch = -atan2f(temp_dir.y, sqrt(temp_dir.x * temp_dir.x + temp_dir.z * temp_dir.z));
static const mat4 identity_mat = mat4(1.0f);
const mat4 rot0_mat = rotate(identity_mat, yaw, vec3(0.0, 1.0, 0.0));
const mat4 rot1_mat = rotate(identity_mat, pitch, vec3(1.0, 0.0, 0.0));
const mat4 translate_mat = translate(identity_mat, temp_dir * displacement);
const vec3 tangent_transformed = normalize((rot0_mat * rot1_mat) * vec4(normalize(geodesic_tangent), 0.0f));
const mat4 rot2_mat = rotate(identity_mat, acos(dot(normalize(geodesic_tangent), tangent_transformed)), vec3(0.0, 0.0, 1.0));
model_mat = translate_mat * rot0_mat * rot1_mat * rot2_mat;
}
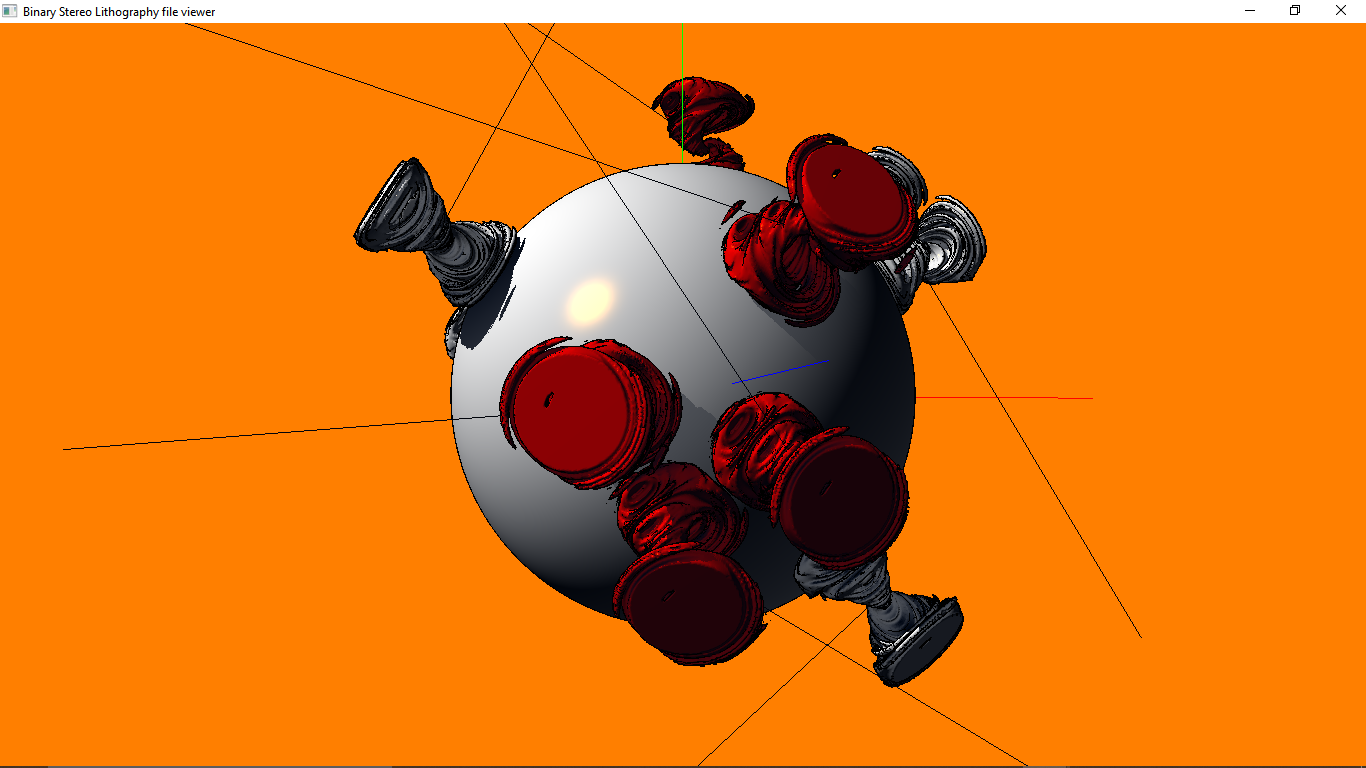