Hi Guys,
I have a basic directional lighting shader that I am working on and all is looking pretty good so far.
I notice on a low poly sphere that you can faintly see lighter areas on the edges of where the triangles are (appearing as quads, as per the geometry).
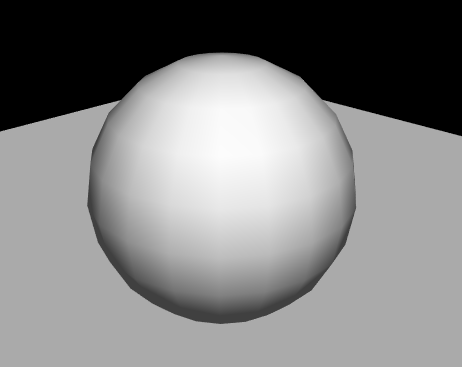
I am wondering why this is and why the shading isn't uniformally smooth across the geometry? The sphere was generated in Maya and looks perfectly fine when rendered within it.
I have attached my shader.
// *** Vertex shader ***
attribute vec3 in_Position;
attribute vec3 in_Normal;
attribute vec2 in_TextureCoord;
varying vec3 v_vNormal;
varying vec3 v_vLightDirection;
void main()
{
// Get object space position
vec4 object_space_pos = vec4( in_Position.x, in_Position.y, in_Position.z, 1.0);
// Translate vertex and pass to fragment shader
gl_Position = gm_Matrices[MATRIX_WORLD_VIEW_PROJECTION] * object_space_pos;
// Normalise the input normals and place into transformed world space
vec4 object_space_normal = gm_Matrices[MATRIX_WORLD] * vec4(normalize(in_Normal), 1.0);
// Pass the world transformed normals to fragment shader
v_vNormal = normalize(object_space_normal.xyz);
// Pass the normalised light position to the fragment shader
// Light direction presently hard coded
v_vLightDirection = normalize(vec3(-5.0, 7.0, -6.0));
}
// *** Fragment shader ***
varying vec3 v_vNormal;
varying vec3 v_vLightDirection;
void main()
{
vec4 diffuse = vec4(1.0, 1.0, 1.0, 1.0);
vec4 ambient = vec4(0.25, 0.25, 0.25, 1.0);
float intensity = max(dot(v_vNormal, v_vLightDirection), 0.0);
vec4 colorOut = max(intensity * diffuse, ambient);
gl_FragColor = colorOut;
}
Is there something I am missing? Or is this just one of those quirky GPU situations?
Also from my understanding, this is a ‘per fragment’ implementation that I have made here, right?
Many thanks in advance.