Heyo, I'm making a custom engine that uses 2:1 isometric sprite graphics with a 3D world simulation. Each render tick, I convert each entity's world position into a screen position, and render their sprite appropriately:
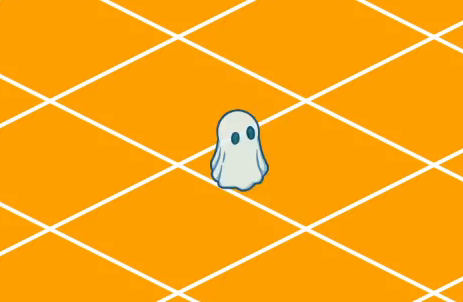
While moving diagonally across the screen, the naive rendering approach (using the raw world -> screen pixel coordinates) caused some jitter. I resolved this by making sure that during diagonal movement, the sprite always gets rendered on the same pixel in the 2:1 pattern. For example, below is a diagonal line with a repeated 2:1 pattern. The first pixel in each step of the pattern is colored blue. If the sprite happened to start its diagonal movement on the first pixel in the pattern, I would continue to round its origin each frame to the closest blue pixel.
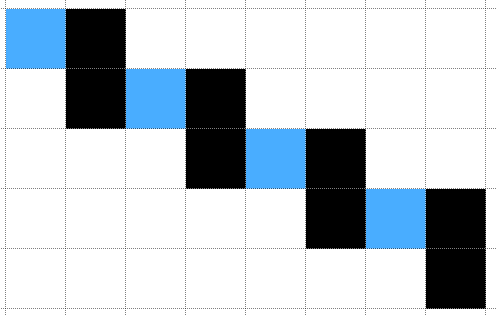
This gives me a sprite that looks like its traveling in a straight line, with no up/down jitter. It introduces a new issue though: if the entity's runspeed doesn't happen to perfectly line up with the pattern, it will sometimes move by 1 step, and sometimes move by 2:
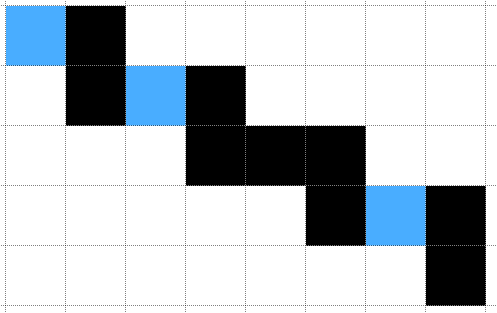
This gives a slight jitter to the graphic, as it moves irregularly across the screen. This jitter isn't present if I choose a runspeed that moves the graphic a consistent number of steps each frame.
My question is: is there any fix for this? Perhaps a different movement algorithm that'll select pixels more optimally? Or a different approach altogether?