I have this code I use to convert a point relative to the camera into a screen coordinate (each axes between 0 and 1, like a texture coordinate):
vec3 CameraPositionToScreenCoord(in vec3 position)
{
float aspect = BufferSize.x / BufferSize.y;
position.x /= position.z / CameraZoom;
position.y /= -position.z / CameraZoom;
position.x /= 2.0f * aspect;
position.y /= 2.0f;
position.xy += 0.5f;
return position;
}
This only works with an orthographic matrix, and as I understand it some VR headsets use funny sheared projection matrices. However, if I do a matrix multiplication of the position times the projection matrix, the results don't seem to be mapped to the screen correctly.
Here is the coordinate visualized using my code above:
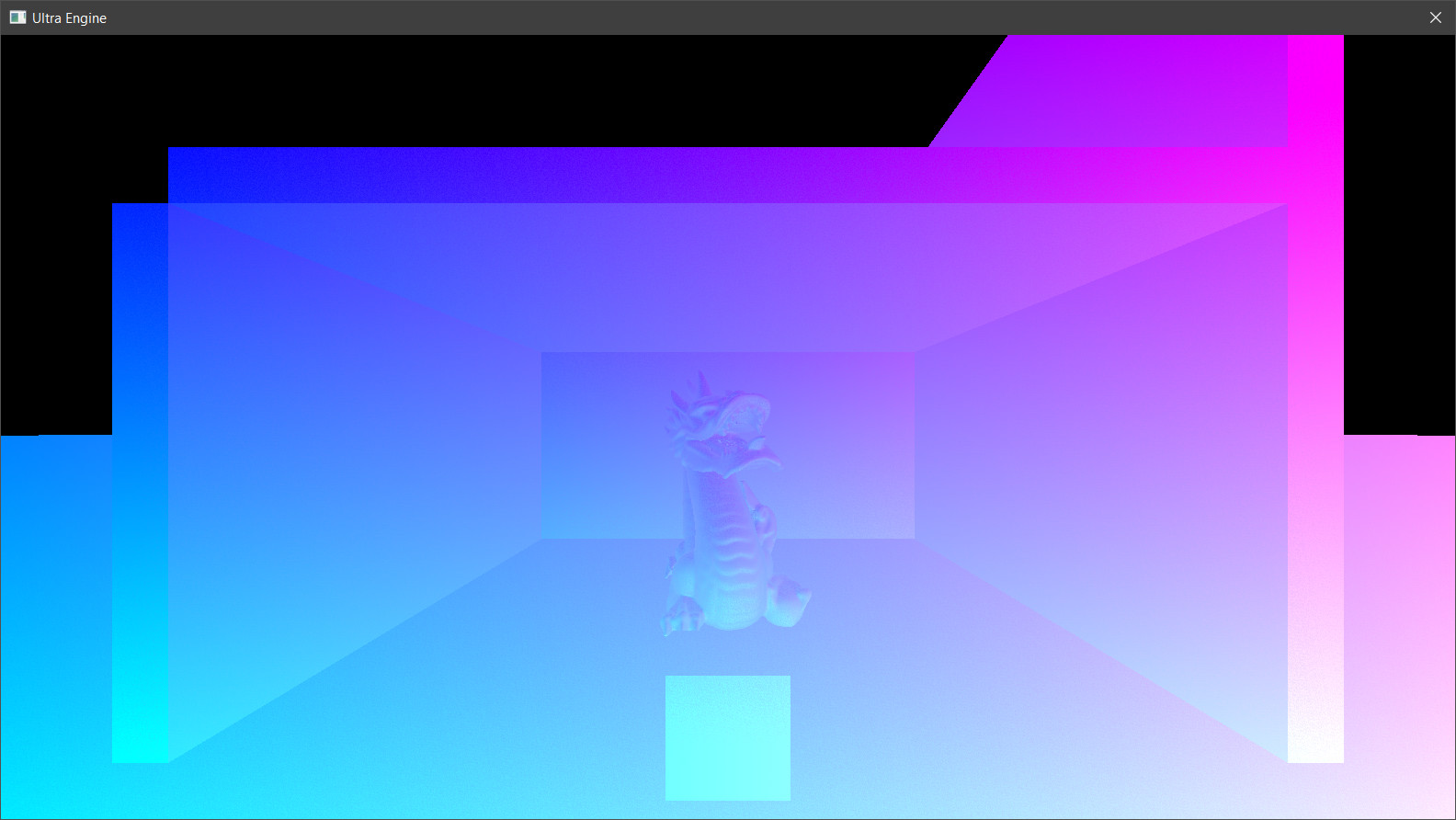
Here is the result if I multiply the position by the projection matrix, just like in the vertex shader:
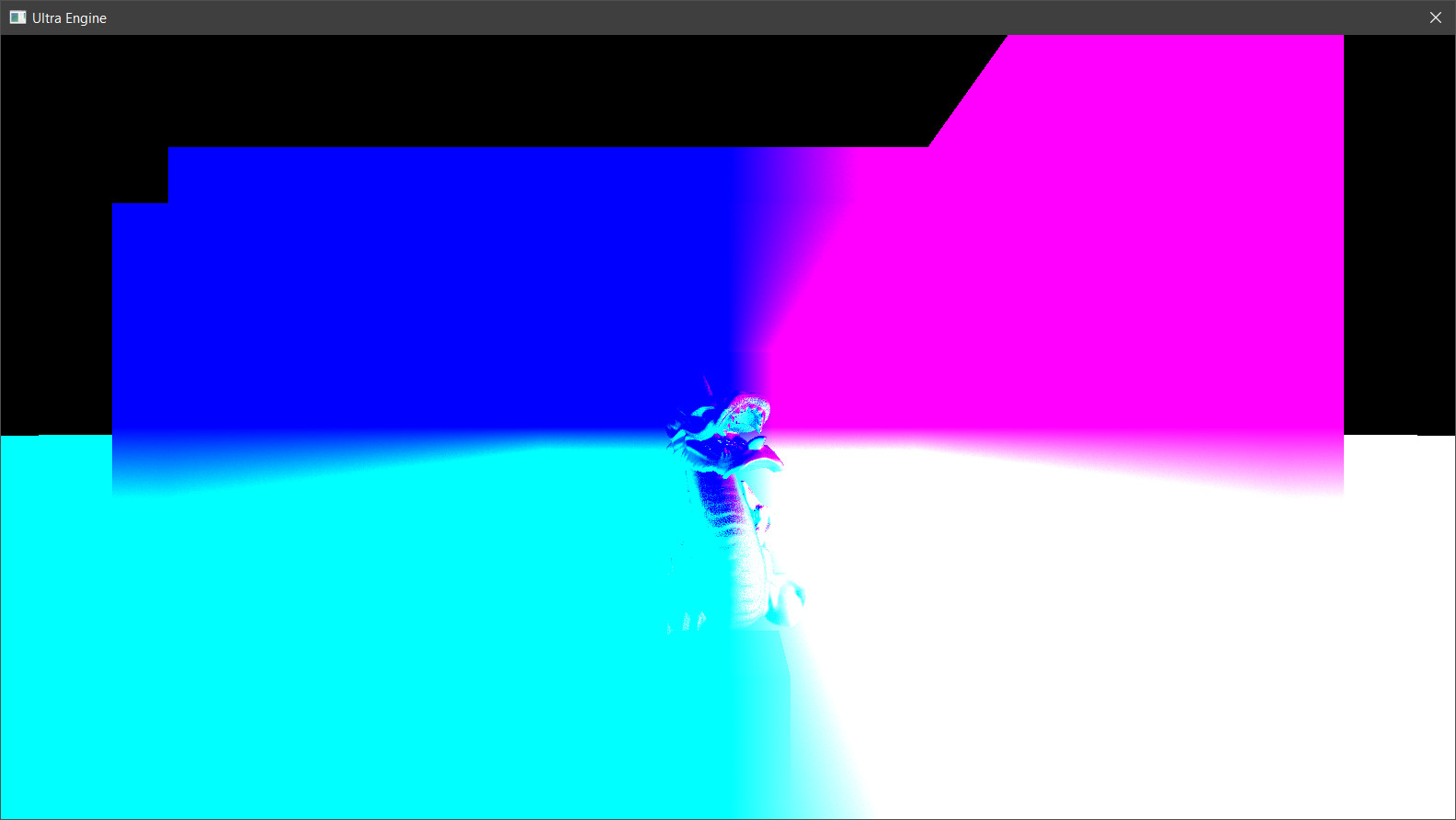
What am I missing here?