Hello, I have an issue I'm trying to solve that I have with blurring.
First here's how I do it:
- Render all normal meshes to render target 1 (which is cleared with alpha 1.0 e.g. not transparent)
- Render all meshes in need of blur to render target 2 (cleared with alpha 0.0, thus has transparency)
- Grab render target 2 and render it to a full screen quad using the gauss blur shader (horizontal/vertical pass) and using render target 3 (also has transparency) to store the final blur buffer
- Bind render target 1 and render fs quad with render target 3 as a texture so it overlays blurred objects on top of normal objects (I also use the same depth target for RT1 and RT2)
Now this is where the issues start.
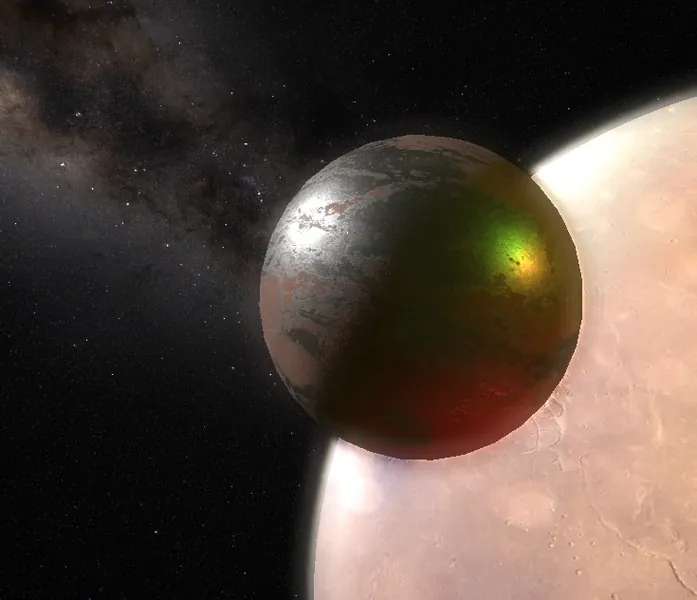
As you can see the normally rendered sphere causes a “gap” halo in a blurred sphere behind it. This happens only after I do the blurring.
This is how RT2 looks before the blur and would fit perfectly in the whole scene, no gaps:
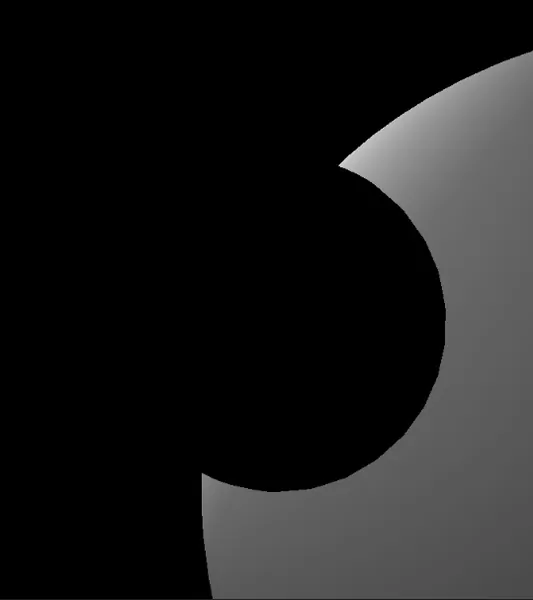
and this is how RT2 looks after the blur:
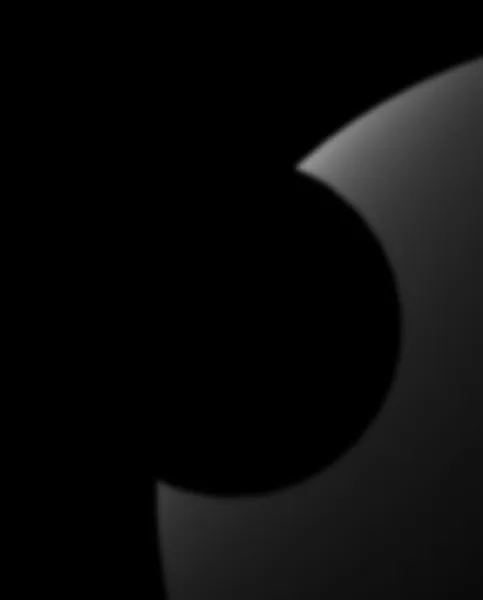
as you can see - the transparent areas spill into the otherwise non-transparent areas adding transparency to edges where it's not needed.
Here's my shader:
cbuffer data : register(b0)
{
float2 dir;
};
cbuffer gauss : register(b2)
{
// 0 = kernel size, 1 - 9 = kernel weights
float gaussData[10];
}
Texture2D tex2D : register(t0);
SamplerState smplr : register(s0);
static const int r = (int)gaussData[0]; // kernel
float4 main(float2 tex : TEXCOORD, float4 screenCoord : SV_POSITION) : SV_TARGET
{
// approximation for 1920x1080
static const float2 pixelStep = { 0.0012, 0.0024 };
// init colors
float4 accColor = tex2D.Sample(smplr, tex) * gaussData[1]; // core pixel weight
// sample horizontal or vertical depending on direction
for (int i = 1; i <= r; i++)
{
accColor += tex2D.Sample(smplr, tex + float2(pixelStep.x * i * dir.x, pixelStep.y * dir.y * i)) * gaussData[i + 1]
+ tex2D.Sample(smplr, tex - float2(pixelStep.x * i * dir.x, pixelStep.y * dir.y * i)) * gaussData[i + 1];
}
return accColor;
}
Can you suggest the solution / improvement?
Kernel weights are generated using the gaussian distribution formula in the program itself and do add up to 1, no matter how many are used.
I also have an additional issue with darker colors spilling onto the normal objects, probably due to blurring resulting in dark grey colors with high enough alpha:
