AhmedSaleh said:
if (triangle_triangle_intersection_check(triB, triA))
Basically you need a function similar to this check, but clipping one triangle into multiple sub triangles, like the pictures show.
But that's too complicated for me to write code out of my head. It's similar to _cg_plane_split_polygon(), but we do not clip with a plane but a triangle, so the intersection becomes a line segment, not just a infinite line. It's thus more complicated. The most complicated case should be this:
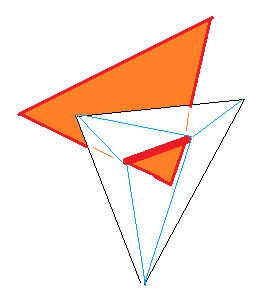
You see the intersection (bold red line) is a segment inside the black triangle. The black triangle becomes 5 new sub-triangles here.
(Some Detail: You also want good tessellation, meaning you want to avoid small angles in triangle corners. This helps to avoid bad triangles and precision issues.)
We then mark the original larger (black) triangle to be inactive (or delete from the list if you prefer), and add the new smaller (blue) triangles to the list.
As we continue to iterate other triangles, those previously added sub-triangles may be split again, and being replaced with even more and smaller sub-sub-triangles.
I can't describe it any better. If there is confusion left, you need to describe in detail which things are unclear.