Hello!
I'm working on a custom Game Engine and I've noticed some odd performance issues when drawing objects with a large amount of triangles that I cannot explain, and I need some tips on how I can narrow the problem down.
I've set up some very simple shaders and a scene with a single object (600k triangles). The object itself is off-screen, i.e. no fragments are being written. I have both an integration for Vulkan and for OpenGL and I've compared the exact same situation between the two. Here are the results:
Vulkan
Baseline (just post-processing, no objects being drawn): 1.63ms per frame
Drawing the object: 1.9ms per frame
These measurements are about what I would expect. Now, in comparison, with my OpenGL implementation:
Baseline: 2.06ms per frame
Drawing the object: 13.2ms per frame!
The baseline framerate is again approximately what I would expect, but clearly there is something fishy going on when the object is being drawn.
I've tried running NVIDIA Nsight to find out what's going on:
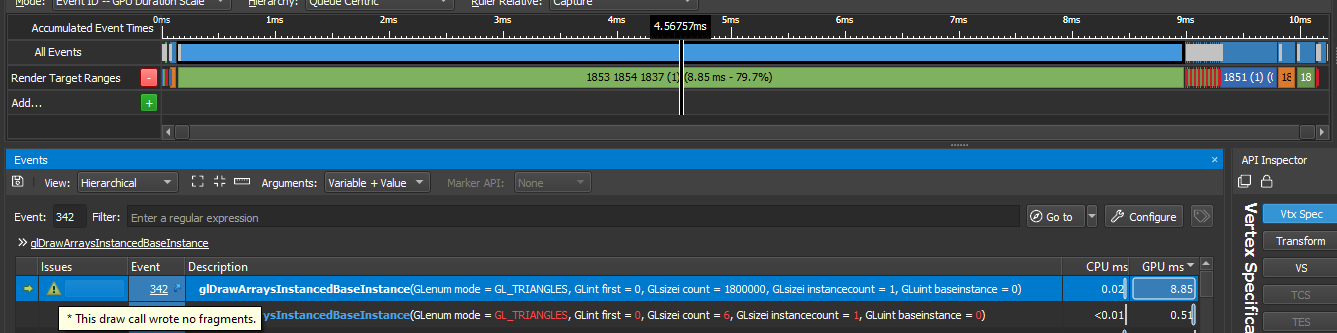
(I've tried using a non-instanced draw call, it made no difference.)
It confirms that:
a) No fragments are actually being drawn
b) The draw-call is definitely the core issue with a whooping 8.85ms
c) It's a GPU bottleneck and not a CPU problem
Here are the shaders themselves:
Vertex shader:
#version 440
#extension GL_ARB_separate_shader_objects : enable
#extension GL_ARB_shading_language_420pack : enable
layout(location = 0) in vec3 in_vert_pos;
void main()
{
gl_Position = vec4(in_vert_pos,1);
}
Fragment shader:
#version 440
#extension GL_ARB_separate_shader_objects : enable
#extension GL_ARB_shading_language_420pack : enable
layout(location = 0) out vec4 fs_color;
void main()
{
fs_color = vec4(1,1,0,1);
}
The C++-code is fairly complex, so posting it here wouldn't do any good.
I have no idea what to even look for, so I'm not sure what other details I could provide, but here's some general information about the OpenGL version and the states:
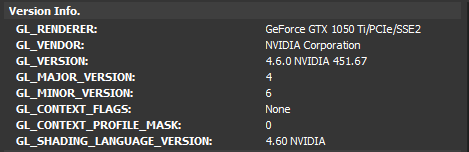
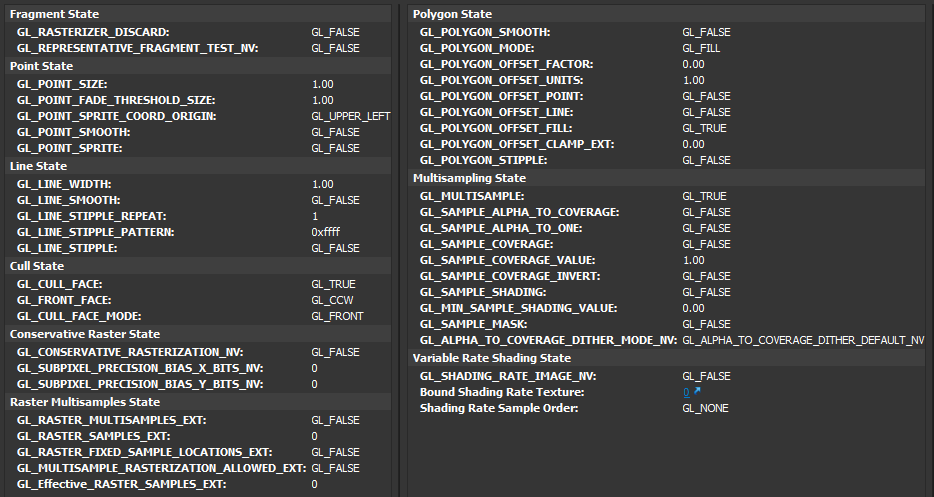
The vertex buffer is created using glCreateBuffers and initialized with glNamedBufferStorage (with the GL_MAP_WRITE_BIT | GL_DYNAMIC_STORAGE_BIT flags). The vertex data is only being written once.
All I need is some hint for what could be causing this issue, so I have an idea what to look for. Thank you!