Hello,
I've tried to implement shadowmapping technique but I am failing to get correct values from shadowmap texture to compare it with current (.z / .w) value.
Here is how I am initializing my shadowmap texture:
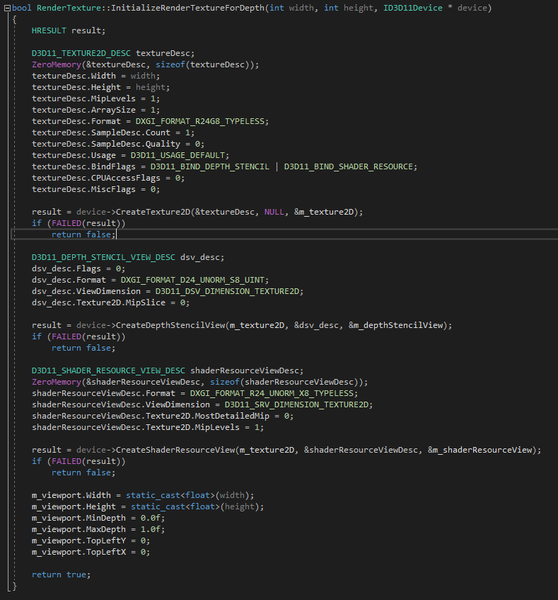
Here is the result:
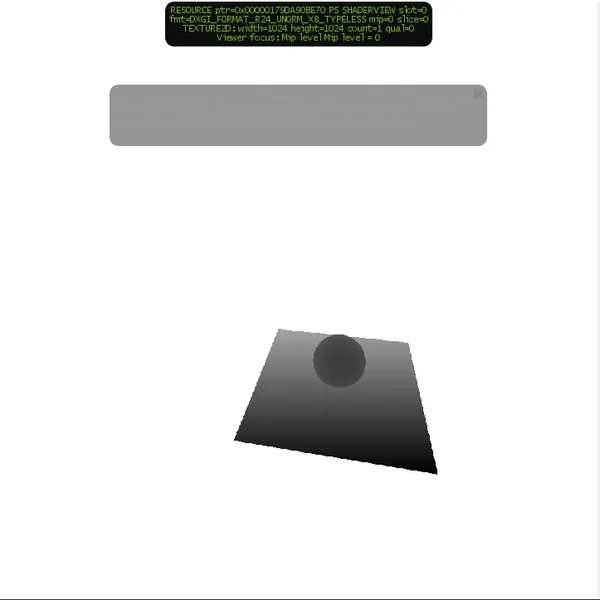
Here is UVs from top of view:
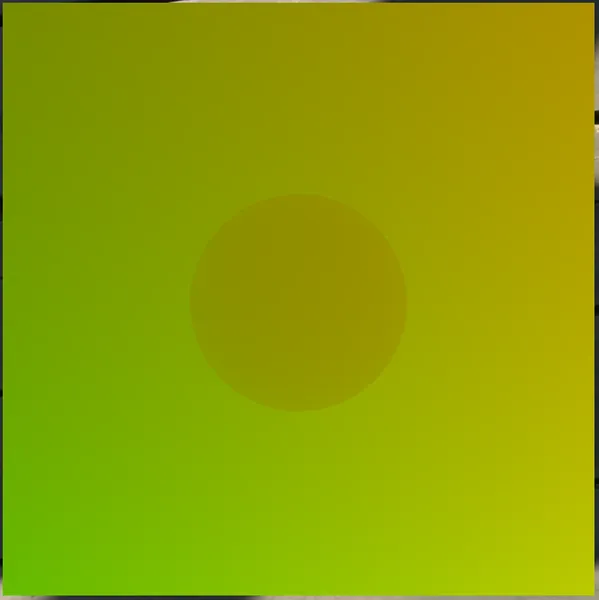
And here is rendered scene with shader code below:
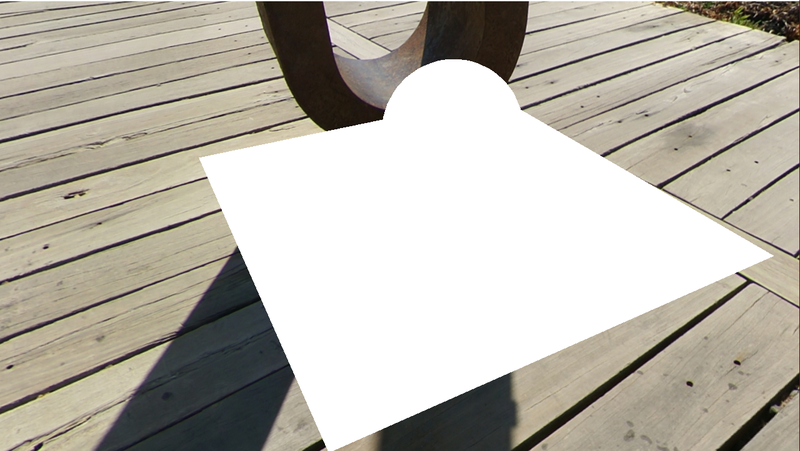
float4 ShadowCalculation(float3 normal, float4 lightPos, float3 pointToLight)
{
const float bias = 0.001f;
float2 projectTexCoord;
projectTexCoord.x = lightPos.x / lightPos.w / 2.0f + 0.5f;
projectTexCoord.y = -lightPos.y / lightPos.w / 2.0f + 0.5f;
if (saturate(projectTexCoord.x) == projectTexCoord.x && saturate(projectTexCoord.y) == projectTexCoord.y)
{
float depthValue = shadowMapTexture.Sample(baseSampler, projectTexCoord.xy).r;
float lightDepthValue = lightPos.z / lightPos.w;
lightDepthValue -= bias;
return depthValue;
}
}
This code is used only to checking projected texture value. UVs presented above are in fact just “projectTexCoord.xy”. Also, they're correct. I was comparing values of UV to determine coordinates in shadowmap texture and it seems fine.
By modifying last line to
return depthValue < 0.99989 ? 0.0 : 1.0;
I am getting different results and I think there might be some clue to be found.
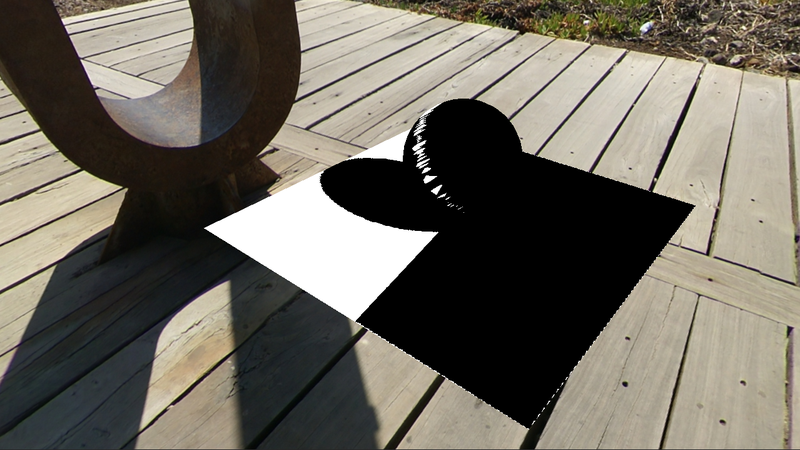