My OpenGL renderer works fine when I do simple forward rendering, but when I try to render to a frame buffer it does not display the last Vertex Array that I tell it to draw. I've changed the order in which they are passed and it always draws all but the last one.
I'm hoping that it's just something silly, like calling something out of order or forgetting to switch a value.
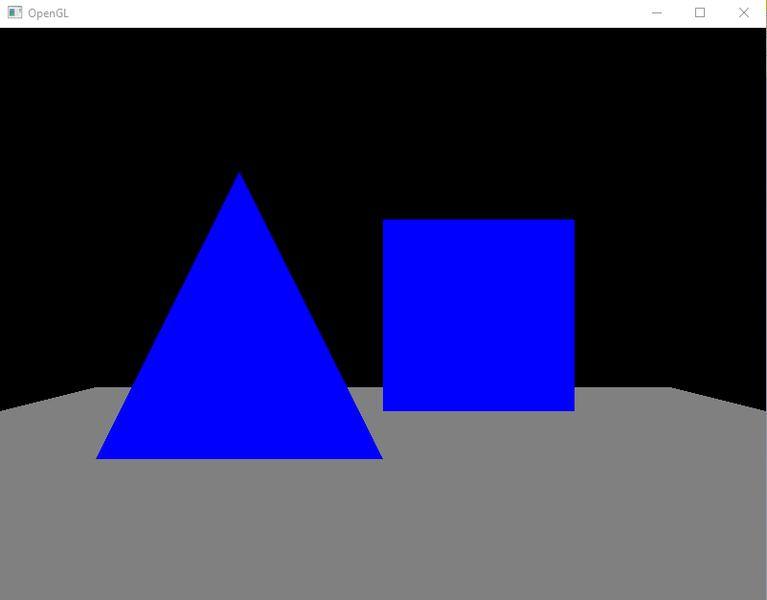
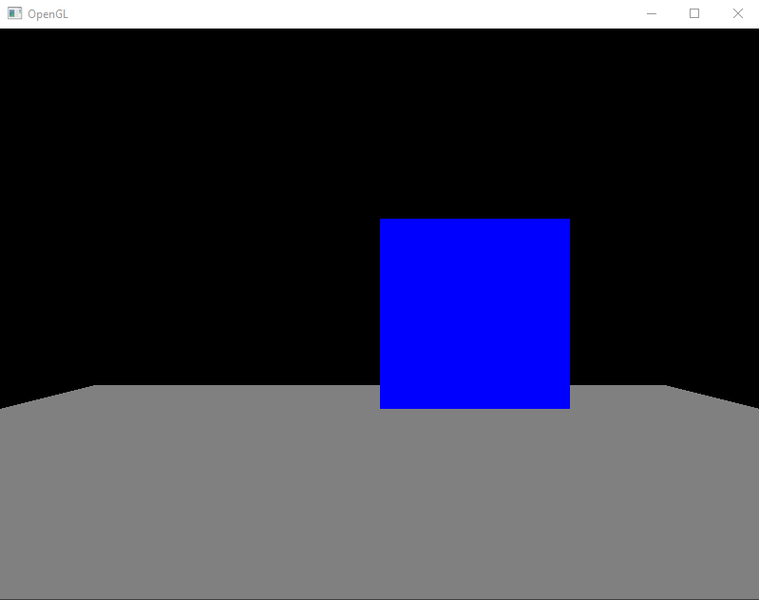
Here's the render code:
void OpenGLRenderer::render(std::vector<RenderObject>& scene, const Camera& camera)
{
glBindFramebuffer(GL_FRAMEBUFFER, camera.targetBuffer); // remove to draw directly to back buffer
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
for (auto currentObject : scene)
{
glUseProgram(currentObject.shader);
glBindVertexArray(currentObject.model);
GLint currentModelSize;
glGetBufferParameteriv(GL_ELEMENT_ARRAY_BUFFER, GL_BUFFER_SIZE, ¤tModelSize);
currentModelSize /= sizeof(GL_UNSIGNED_INT);
GLuint transformLocation = glGetUniformLocation(currentObject.shader, "transform");
glm::mat4 mvp = camera.projection * camera.view * currentObject.transform;
glUniformMatrix4fv(transformLocation, 1, GL_FALSE, glm::value_ptr(mvp));
glDrawElements(GL_TRIANGLES, currentModelSize, GL_UNSIGNED_INT, (void*)0);
}
glBindFramebuffer(GL_FRAMEBUFFER, 0);
}
and the main run loop:
while (isRunning)
{
getInput();
renderer.render(scene, camera);
renderer.drawBufferToWindow(outputFrameBuffer.textureId, 800, 600); // remove to draw directly to backbuffer
glfwSwapBuffers(window);
}
the function do draw the framebuffer to the screen:
void OpenGLRenderer::drawBufferToWindow(const GLuint textureToRender, const unsigned int windowWidth, const unsigned int windowHeight)
{
glBindFramebuffer(GL_FRAMEBUFFER, 0); // set default (window) render target
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glViewport(0, 0, windowWidth, windowHeight);
glEnableVertexAttribArray(0); // make sure no other arrays are bound
glBindBuffer(GL_ARRAY_BUFFER, windowRenderPoly);
glVertexAttribPointer(0, 2, GL_FLOAT, GL_FALSE, 0, (void*)0);
glBindTexture(GL_TEXTURE_2D, textureToRender);
glUseProgram(windowRenderShader);
glDrawArrays(GL_TRIANGLES, 0, 3);
glDisableVertexAttribArray(0);
}
finally the renderObject class:
class RenderObject
{
public:
unsigned int shader;
unsigned int model;
glm::mat4 transform;
};
I also found that if I remove the glClear command in the main render function it draws everything, even when drawing to the frame buffer. Obviously it can't actually be used this way though, as it leaves artifacts from previous frames.