Alright so I've localized the issue. It happens when I compile the hull shader with the optimization flag turned on - if I disable it, it works perfectly fine ?
All other shaders are compiled with optimization flag and they work fine. Looking at the diff between the textfiles I find some things like this-
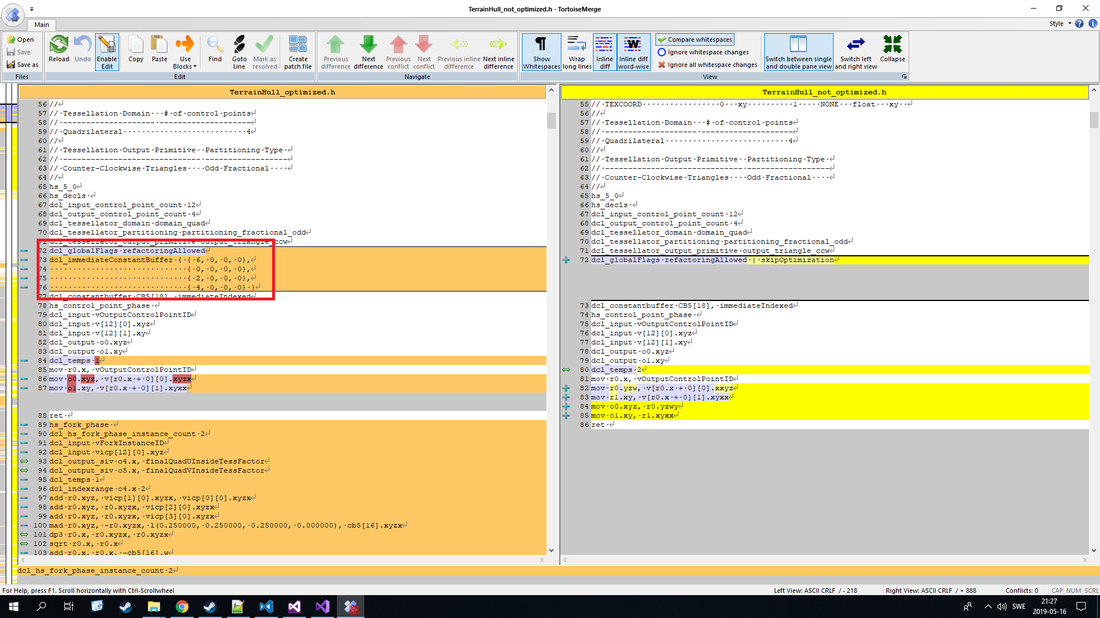
What is this immediate constant buffer? What are these values inside?
I'm attaching the full shader code aswell if it helps:
#ifndef TERRAIN_HULL_HLSL
#define TERRAIN_HULL_HLSL
#include "TerrainCommon.hlsl"
float3 ComputePatchMidpoint( float3 corner1, float3 corner2, float3 corner3, float3 corner4 )
{
return ( corner1 + corner2 + corner3 + corner4 ) / 4.0f;
}
float CalculateTessellationfactor( float3 worldPatchMidpoint )
{
float cameraToPatchDistance = distance( gWorldEyePos, worldPatchMidpoint );
float scaledDistance = ( cameraToPatchDistance - gMinZ ) / ( gMaxZ - gMinZ );
scaledDistance = clamp( scaledDistance, 0.0f, 1.0f );
return pow( 2, lerp( 6.0f, 2.0f, scaledDistance ) );
}
PatchTess PatchHS( InputPatch<VertexOut, 12> inputVertices )
{
PatchTess patch;
float3 midPatchMidpoint = ComputePatchMidpoint( inputVertices[ 0 ].mWorldPosition, inputVertices[ 1 ].mWorldPosition, inputVertices[ 2 ].mWorldPosition, inputVertices[ 3 ].mWorldPosition );
float3 edgePatchMidpoint[] = {
ComputePatchMidpoint( inputVertices[ 0 ].mWorldPosition, inputVertices[ 1 ].mWorldPosition, inputVertices[ 4 ].mWorldPosition, inputVertices[ 5 ].mWorldPosition ),
ComputePatchMidpoint( inputVertices[ 1 ].mWorldPosition, inputVertices[ 2 ].mWorldPosition, inputVertices[ 6 ].mWorldPosition, inputVertices[ 7 ].mWorldPosition ),
ComputePatchMidpoint( inputVertices[ 2 ].mWorldPosition, inputVertices[ 3 ].mWorldPosition, inputVertices[ 8 ].mWorldPosition, inputVertices[ 9 ].mWorldPosition ),
ComputePatchMidpoint( inputVertices[ 3 ].mWorldPosition, inputVertices[ 0 ].mWorldPosition, inputVertices[ 10 ].mWorldPosition, inputVertices[ 11 ].mWorldPosition )
};
float midPatchTessFactor = CalculateTessellationfactor( midPatchMidpoint );
float edgePatchTessFactors[] = {
CalculateTessellationfactor( edgePatchMidpoint[ 3 ] ),
CalculateTessellationfactor( edgePatchMidpoint[ 0 ] ),
CalculateTessellationfactor( edgePatchMidpoint[ 1 ] ),
CalculateTessellationfactor( edgePatchMidpoint[ 2 ] )
};
patch.mEdgeTess[ 0 ] = min( midPatchTessFactor, edgePatchTessFactors[ 0 ] );
patch.mEdgeTess[ 1 ] = min( midPatchTessFactor, edgePatchTessFactors[ 1 ] );
patch.mEdgeTess[ 2 ] = min( midPatchTessFactor, edgePatchTessFactors[ 2 ] );
patch.mEdgeTess[ 3 ] = min( midPatchTessFactor, edgePatchTessFactors[ 3 ] );
patch.mInsideTess[ 0 ] = midPatchTessFactor;
patch.mInsideTess[ 1 ] = midPatchTessFactor;
return patch;
}
[domain("quad")]
[partitioning("fractional_odd")]
[outputtopology("triangle_ccw")]
[outputcontrolpoints(4)]
[patchconstantfunc("PatchHS")]
HullOut hs_main( InputPatch<VertexOut, 12> verticeData, uint index : SV_OutputControlPointID )
{
HullOut ret;
ret.mWorldPosition = verticeData[ index ].mWorldPosition;
ret.mTexcoord = verticeData[ index ].mTexcoord;
return ret;
}
#endif
#ifndef TERRAIN_COMMON_HLSL
#define TERRAIN_COMMON_HLSL
#include "Common.hlsl"
// TODO: move to common perframe CB
cbuffer PerFrameConstants : register(CBUFFER_REGISTER_DOMAIN)
{
float gMinDistance;
float gMaxDistance;
float gMinFactor;
float gMaxFactor;
}
cbuffer PerTerrainConstants : register(CBUFFER_REGISTER_EXTRA)
{
float gHeightModifier;
float gVariationScale;
float gWorldMinX;
float gWorldMinZ;
float gWorldMaxX;
float gWorldMaxZ;
}
struct PatchTess
{
float mEdgeTess[4] : SV_TessFactor;
float mInsideTess[2] : SV_InsideTessFactor;
};
struct VertexOut
{
float3 mWorldPosition : POSITION;
float2 mTexcoord : TEXCOORD;
};
struct DomainOut
{
float4 mPosition : SV_POSITION;
float3 mNormal : NORMAL;
float2 mTexcoord : TEXCOORD;
};
struct HullOut
{
float3 mWorldPosition : POSITION;
float2 mTexcoord : TEXCOORD;
};
#endif
#ifndef CONSTANTS_HLSL
#define CONSTANTS_HLSL
#define CBUFFER_SLOT_VERTEX 0
#define CBUFFER_SLOT_PIXEL 1
#define CBUFFER_SLOT_COMPUTE 2
#define CBUFFER_SLOT_DOMAIN 3
#define CBUFFER_SLOT_HULL 4
#define CBUFFER_SLOT_PER_FRAME 5
#define CBUFFER_SLOT_EXTRA 6
#define CBUFFER_REGISTER_VERTEX b0
#define CBUFFER_REGISTER_PIXEL b1
#define CBUFFER_REGISTER_COMPUTE b2
#define CBUFFER_REGISTER_DOMAIN b3
#define CBUFFER_REGISTER_HULL b4
#define CBUFFER_REGISTER_PER_FRAME b5
#define CBUFFER_REGISTER_EXTRA b6
#define SAMPLER_SLOT_ANISOTROPIC 0
#define SAMPLER_SLOT_POINT 1
#define SAMPLER_SLOT_POINT_COMPARE 2
#define SAMPLER_SLOT_LINEAR 3
#define SAMPLER_SLOT_LINEAR_WRAP 4
#define SAMPLER_REGISTER_ANISOTROPIC s0
#define SAMPLER_REGISTER_POINT s1
#define SAMPLER_REGISTER_POINT_COMPARE s2
#define SAMPLER_REGISTER_LINEAR s3
#define SAMPLER_REGISTER_LINEAR_WRAP s4
#define TEXTURE_SLOT_DIFFUSE 0
#define TEXTURE_SLOT_NORMAL 1
#define TEXTURE_SLOT_DEPTH 2
#define TEXTURE_SLOT_PERLIN 3
#define TEXTURE_SLOT_EXTRA 4
#define SBUFFER_SLOT_BONE_TRANSFORMS 4
#define SBUFFER_SLOT_EXTRA 5
#define TEXTURE_REGISTER_DIFFUSE t0
#define TEXTURE_REGISTER_NORMAL t1
#define TEXTURE_REGISTER_DEPTH t2
#define TEXTURE_REGISTER_PERLIN t3
#define TEXTURE_REGISTER_EXTRA t4
#define SBUFFER_REGISTER_BONE_TRANSFORMS t4
#define SBUFFER_REGISTER_EXTRA t5
#define UAV_SLOT 0
#define UAV_REGISTER u0
#define SDSM_THREAD_GROUP_SIZE 16
#define SDSM_NUM_THREADS (SDSM_THREAD_GROUP_SIZE * SDSM_THREAD_GROUP_SIZE)
#define NUM_BONES_PER_VERTEX 4
#define LUM_MAP_WIDTH 1024
#define LUM_MAP_HEIGHT 1024
#endif
I've attached both compiled shaders - is there something obvious wrong?
TerrainHull_not_optimized.h
TerrainHull_optimized.h