@Scouting Ninja @Cold.bo @Mike2343
I have progressed a bit with the project -
I have randomly generated spheres having different alphabets and predefined words.
Presently, I cannot compare the alphabet in the sphere with the alphabets of the word.
Let me show you the code
For randomly generating different alphabets -
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class GenerateRandom : MonoBehaviour {
private Text[] Circle;
// Use this for initialization
void Start () {
Circle = GameObject.FindGameObjectWithTag ("Text").GetComponentsInChildren<Text> ();
char[] S = "qwertyuiopasdfghjklzxcvbnm".ToCharArray ();
for (int i = 0; i < 3; i++) {
Circle .text = S [Random.Range (0, 25)].ToString ();
}
}
// Update is called once per frame
void Update () {
}
}
For Randomly generating a word -
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class RandomWord : MonoBehaviour {
string[] Words = { "great", "stage", "peak", "street", "please" };
private Text texter;
// Use this for initialization
void Start () {
texter = GameObject.FindGameObjectWithTag ("Sphere").GetComponent<Text>();
texter.text = Words[Random.Range (0, 4)].ToString();
}
// Update is called once per frame
void Update () {
}
}
For collision detection and checking the correct alphabet -
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Destroy : MonoBehaviour {
private char[] Check;
private string Me,You;
// Use this for initialization
void Start () {
Me = GameObject.FindGameObjectWithTag ("Sphere").GetComponent<Text> ().ToString ();
}
// Update is called once per frame
void Update () {
}
void OnCollisionEnter2D(Collision2D other)
{
int Length = Me.Length;
Check = Me.ToCharArray ();
Debug.Log ("Collision");
if(GameObject.FindWithTag ("Background"))
{
You = other.gameObject.GetComponent<Text> ().ToString ();
if(You != null)
{
for(int i=0 ; i<Length; i++)
{
if (You == Check .ToString ())
Debug.Log ("Matched");
}
}
}
Destroy (other.gameObject);
}
}
Now I am getting the issue in the picture I have shared.
When I double click the error, it redirects me to -
You = other.gameObject.GetComponent<Text> ().ToString ();
Looking forward to your help
Thanks
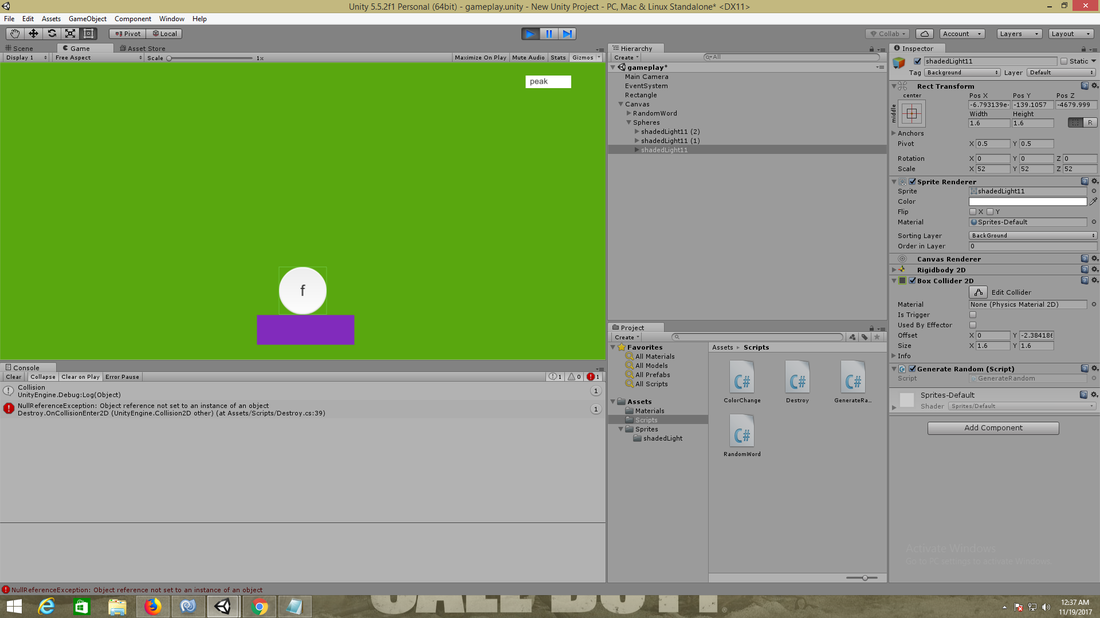