Hi,
I'm making a DirectX/OpenGL engine for learning purposes. However I'm having a problem with my implementation of the rotation matrix for DirectX.
The rotation on the x and z axis looks fine for both DirectX and OpenGL. Also the rotation on the Y axis in OpenGL looks fine too. However the rotation on the Y axis in DirectX looks very weird.
This how OpenGL Y axis rotation looks like. Which looks perfectly fine.
And here how DirectX Y axis rotation looks like.
The triangle in DirectX looks like a right-angled triangle for some reason.
Now I understand why the triangle doesn't get rendered in DirectX when it is flipped. That is not the problem, the problem is that the triangle looks like it somehow turned into a right-angled triangle. I don't know why.
This is the image I'm using for my implementation.
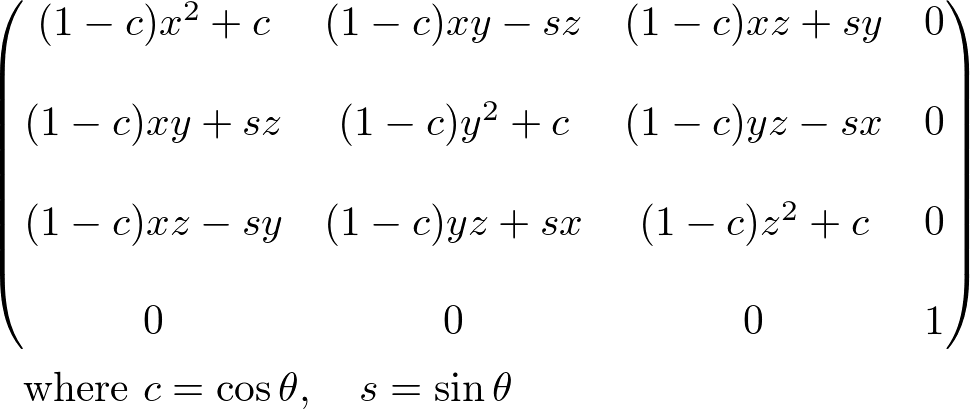
Here is my matrix function
/*
m11 m12 m13 m14 x
m21 m22 m23 m24 y
m31 m32 m33 m34 z
x y z w m41 m42 m43 m44 w
C++ array indices [0, 1, 2, 3 ], [4, 5, 6, 7 ], [8, 9, 10, 11 ] [12, 13, 14, 15 ]
DirectX matrix layout [m11, m21, m31, m41], [m12, m22, m32, m42], [m13, m23, m33, m43], [m14, m24, m34, m44]
OpenGL matrix layout [m11, m12, m13, m14], [m21, m22, m23, m24], [m31, m32, m33, m34], [m41, m42, m43, m44]
*/
const int ROW_COLUMN_SIZE = 4;
Matrix4 Matrix4::Rotate(const float &angle, const Vector3 &axis)
{
Matrix4 RotationMatrix = Matrix4::Identity();
float r = ToRadians(angle);
float c = cos(r);
float s = sin(r);
float oc = 1 - c;
if (Graphics::Renderer::renderingAPI == Graphics::Renderer::RenderingAPI::DirectX11)
{
//First column
RotationMatrix.elements[0 * ROW_COLUMN_SIZE + 0] = axis.x * axis.x * oc + c;
RotationMatrix.elements[0 * ROW_COLUMN_SIZE + 1] = axis.x * axis.y * oc + axis.z * s;
RotationMatrix.elements[0 * ROW_COLUMN_SIZE + 2] = axis.x * axis.z * oc - axis.y * s;
//Second column
RotationMatrix.elements[1 * ROW_COLUMN_SIZE + 0] = axis.x * axis.y * oc - axis.z * s;
RotationMatrix.elements[1 * ROW_COLUMN_SIZE + 1] = axis.y * axis.y * oc + c;
RotationMatrix.elements[1 * ROW_COLUMN_SIZE + 2] = axis.y * axis.z * oc + axis.x * s;
//Third column
RotationMatrix.elements[2 * ROW_COLUMN_SIZE + 0] = axis.x * axis.z * oc + axis.y * s;
RotationMatrix.elements[2 * ROW_COLUMN_SIZE + 1] = axis.y * axis.z * oc - axis.x * s;
RotationMatrix.elements[2 * ROW_COLUMN_SIZE + 2] = axis.z * axis.z * oc + c;
}
else if (Graphics::Renderer::renderingAPI == Graphics::Renderer::RenderingAPI::OpenGL4_5)
{
//First row
RotationMatrix.elements[0 + 0 * ROW_COLUMN_SIZE] = axis.x * axis.x * oc + c;
RotationMatrix.elements[1 + 0 * ROW_COLUMN_SIZE] = axis.x * axis.y * oc - axis.z * s;
RotationMatrix.elements[2 + 0 * ROW_COLUMN_SIZE] = axis.x * axis.z * oc + axis.y * s;
//Second row
RotationMatrix.elements[0 + 1 * ROW_COLUMN_SIZE] = axis.x * axis.y * oc + axis.z * s;
RotationMatrix.elements[1 + 1 * ROW_COLUMN_SIZE] = axis.y * axis.y * oc + c;
RotationMatrix.elements[2 + 1 * ROW_COLUMN_SIZE] = axis.y * axis.z * oc - axis.x * s;
//Third row
RotationMatrix.elements[0 + 2 * ROW_COLUMN_SIZE] = axis.x * axis.z * oc - axis.y * s;
RotationMatrix.elements[1 + 2 * ROW_COLUMN_SIZE] = axis.y * axis.z * oc + axis.x * s;
RotationMatrix.elements[2 + 2 * ROW_COLUMN_SIZE] = axis.z * axis.z * oc + c;
}
return RotationMatrix;
}
OpenGL and DirectX rotation
[spoiler]
OpenGL rotation
X axis
Y axis
Z axis
DirectX rotation
X axis
Y axis
Z axis