I'm trying to figure out if there exists a clever way to test if a frustum is visible in a frustum. Not even a generic way - I'm using camera frustums to be precise - each is defined by 6 planes and 8 corner points. It has to consider both cases - orthographic and perspective cases (i.e. it can't be generalized to be just OBB in either case)
My first idea was to use generic 3D SAT, which works, but that really seems like "using a nuke on an ant" ... with all of its costs. So I'm trying to figure out if there is a more clever way.
I'm considering using same approach as with AABBs - it is just going to be slightly more expensive. So the situation is like this:

To simplify the case - I sketched this in 2D (thanks Geogebra!), there are 2 frustums GHJI and OPRQ. As frustums are convex objects, the approach could be the same as is used with boxes in general (testing whether longest line along normal in the second frustum is inside the testing plane, intersects it or is outside), i.e.:
result = INSIDE
for (i = 0; i < 6; i++)
{
if (distance(plane[i], maxPoint) < 0)
{
return OUTSIDE
}
else if (distance(plane[i], minPoint) < 0)
{
result = INTERSECT
}
}
return result
Where minPoint and maxPoint are the points from other frustum sorted by distance to plane (negative distance meaning point being ‘behind’ the plane). When looking at in in the above scenario it yields:
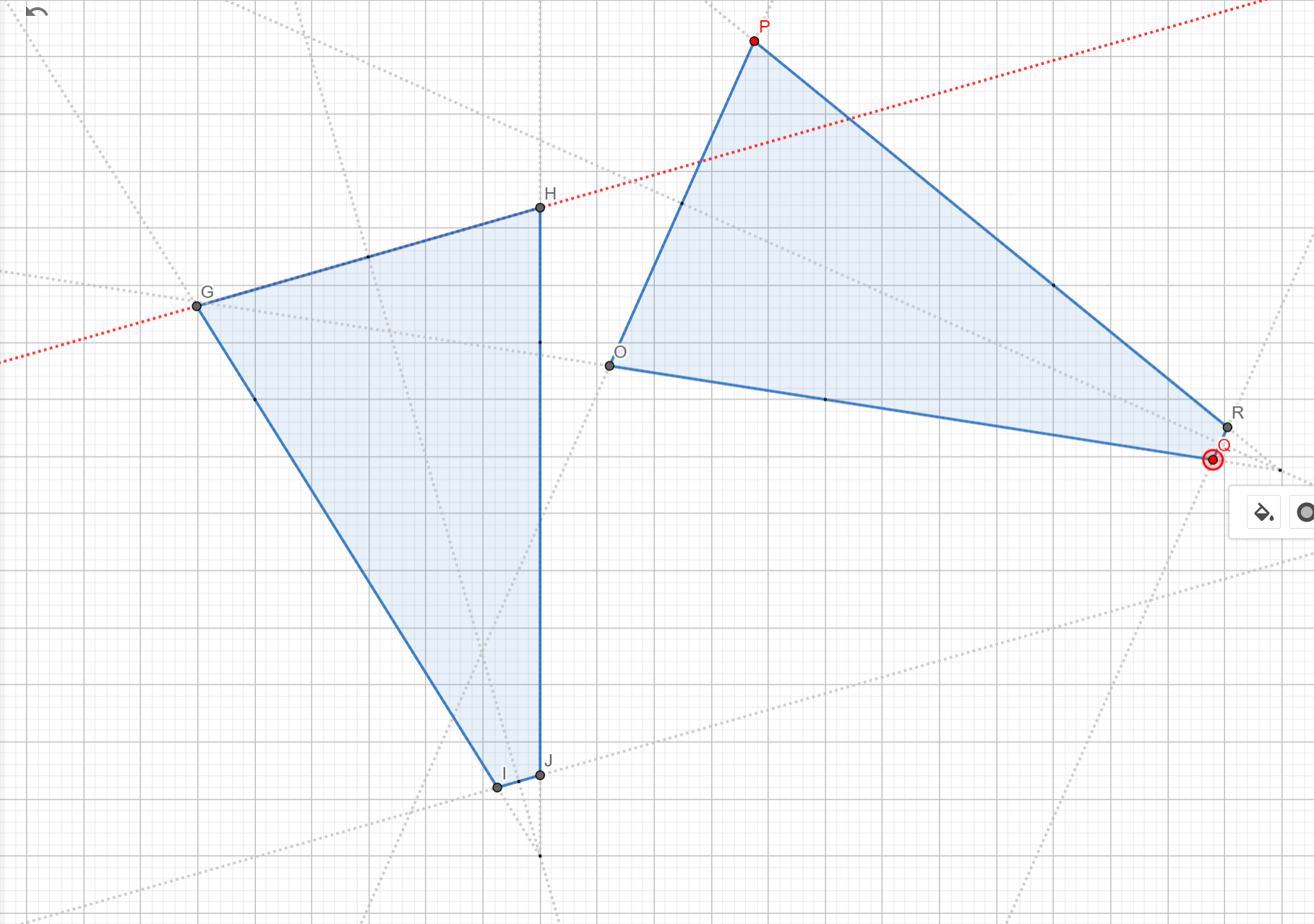
For the first plane result will hold INTERSECT.
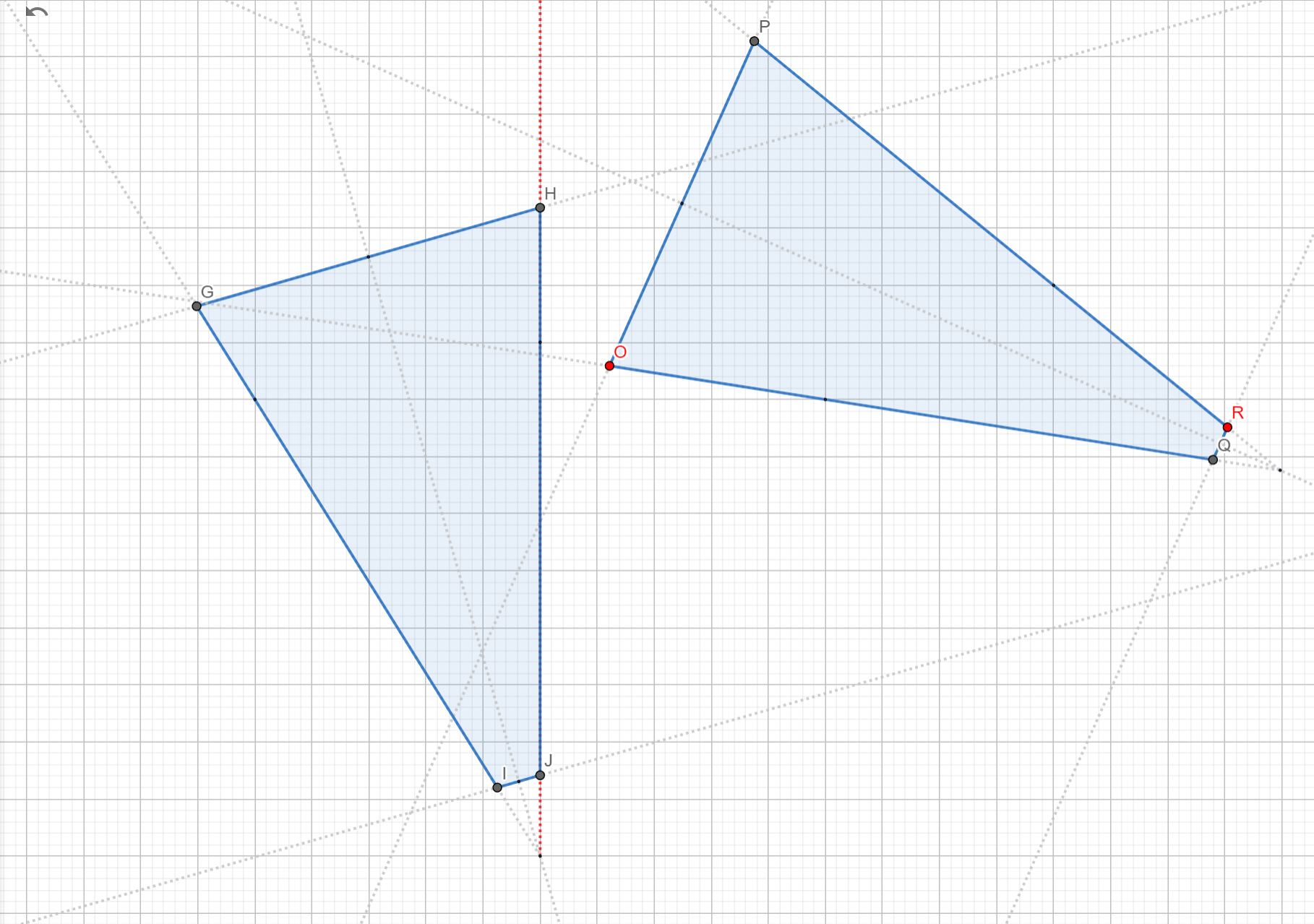
For the second plane it will straight return OUTSIDE.
I did the similar approach for the intersecting frustums, and it seems to work properly.
The only detail (compared to AABB test) is that one has to compute distance to all 8 points of frustum (and can't use axes for optimization) to select minPoint and maxPoint.
To the question - is my approach here correct, or am I missing some case where this test will fail?