I am trying to implement a BC5 decompressor in C++ using this C# code as a guide:
https://github.com/yretenai/bcffnet/blob/develop/BCFF/BlockDecompressor.cs
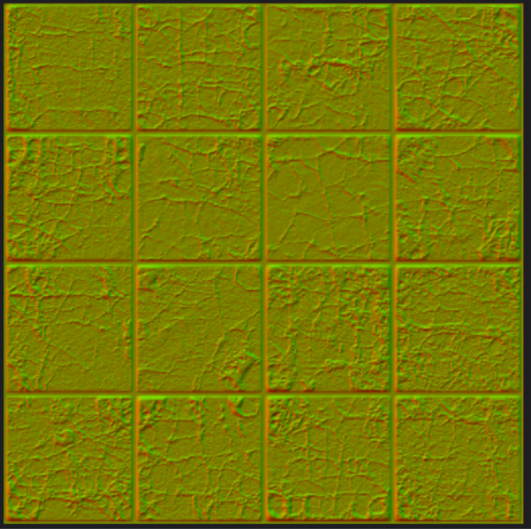
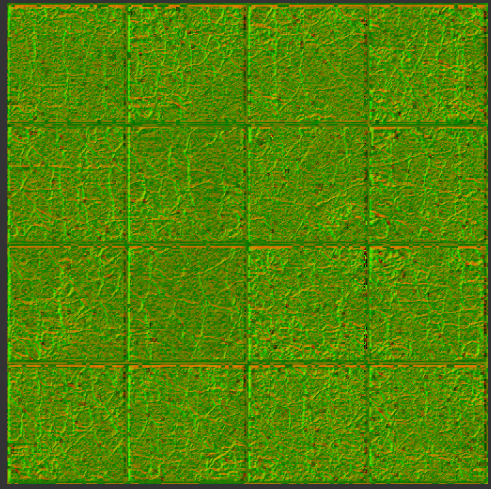
Any ideas what is wrong? Here is my decompress block code:
float GetRed(int Red0, int Red1, unsigned char Index)
{
if (Index == 0)
{
return float(Red0) / 255.0f;
}
if (Index == 1)
{
return float(Red1) / 255.0f;
}
float Red0f = float(Red0) / 255.0f;
float Red1f = float(Red1) / 255.0f;
if (Red0 > Red1)
{
Index -= 1;
return (Red0f * float(7 - Index) + Red1f * float(Index)) / 7.0f;
}
else
{
if (Index == 6)
{
return 0.0f;
}
if (Index == 7)
{
return 1.0f;
}
Index -= 1;
return (Red0f * float(5 - Index) + Red1f * float(Index)) / 5.0f;
}
}
unsigned char GetIndex(uint64_t strip, int offset)
{
return (unsigned char)((strip >> (3 * offset + 16)) & 0x7);
}
void BC5ReadBlock(uint64_t strip, std::array<float, 16>& block)
{
unsigned char Red0, Red1;
memcpy(&Red0, &strip, 1);
memcpy(&Red1, &strip + 1, 1);
for (int i = 0; i < 16; ++i)
{
int index = GetIndex(strip, i);
block[i] = GetRed(Red0, Red1, index);
}
}
Texture loading code: float r, g;
std::array<float, 16> rblock, gblock;
uint64_t strip;
for (x = 0; x < blocks.x; ++x)
{
for (y = 0; y < blocks.y; ++y)
{
stream->Read(&strip, 8);
BC5::BC5ReadBlock(strip, rblock);
stream->Read(&strip, 8);
BC5::BC5ReadBlock(strip, gblock);
int index = 0;
for (int bx = 0; bx < 4; bx++)
{
for (int by = 0; by < 4; by++)
{
pixmap->WritePixel(x * 4 + bx, y * 4 + by, RGBA(Clamp(Floor(rblock[index] * 255.0f),0,255), Clamp(Floor(gblock[index] * 255.0f),0,255), 0, 255));
index++;
}
}
}
}