I am trying to prevent this problem in opengl:
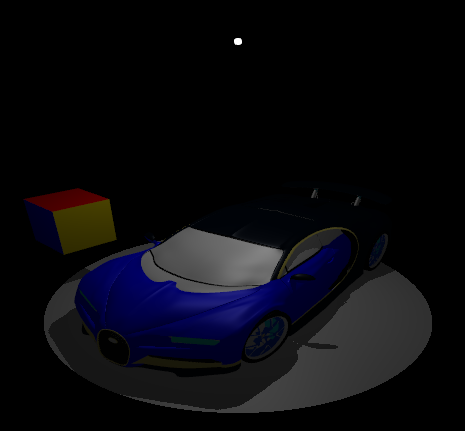
as you can see there is hard fall of in light if a fragment is too far away from light.
this was when the light sphere radius was 11
I then mildly solved this problem and now its like this:
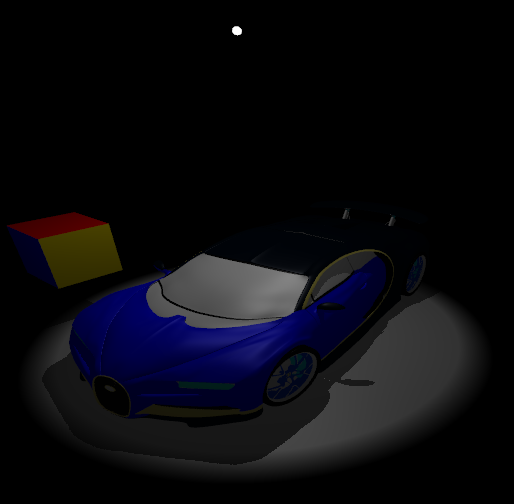
this was done with this code:
for (int i = 0; i < activePointLights; i++) {
float dist=distance(f_position,pointLights[i].position);
if (dist <= pointLights[i].radius+pointLights[i].dropoffRadius){
result += CalcPointLight(pointLights[i], norm, f_position, viewDir, diffColor, tangentLightPos, tangentFragPos)*clamp(2*pointLights[i].dropoffRadius*(1-dist)+pointLights[i].radius-pointLights[i].dropoffRadius,0,1);
}
}
here dropoffradius was 1 and the light sphere radius was 23
the equation inside clamp was made by linearly interpolating input from (sphereradius - dropoffradius to sphereradius + dropoffradius)
being mapped to range (1 to 0)
.
When I increase pointLights[i].dropoffRadius
to 2 I get way less illuminated area and I have to crank up the radius of the pointlight sphere.
what I want now is to make the edges of the boundary of light affecting fragments in plane of above picture more soft controlled by a value like pointLights[i].dropoffRadius
.