Hello! I am making a small font engine for draw statistics on screen. My example:
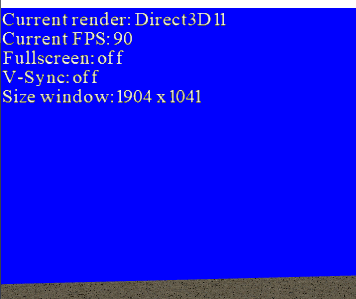
My system is worked so:
- I create dynamic vertex buffer with size float3 (position) x float2 (uv coordinates) x 1000
- Start game loop
- For every frame I map vertex buffer (font engine)
- I update necessary vertex (fps etc)
- Unmap vertex buffer (font engine)
- Draw vertex buffer (font engine) (1 draw call)
I am kind of a beginner (many optimization concepts are not familiar to me), so I wanted to know:
- Is this way of drawing text effective? After all, the constant display of memory, if I'm not mistaken, is very expensive. For example OpenGL prints warning:
Debug message (131186): Buffer performance warning: Buffer object 1 (bound to GL_VERTEX_ATTRIB_ARRAY_BUFFER_BINDING_ARB (0), GL_VERTEX_ATTRIB_ARRAY_BUFFER_BINDING_ARB (1), and GL_ARRAY_BUFFER_ARB, usage hint is GL_DYNAMIC_DRAW) is being copied / moved from VIDEO memory to DMA CACHED memory.
Source: API
Type: Performance
Severity: medium
2. I noticed that when I switch to full screen, I have FPS losses (~ 150 - 200). Is this normal practice, or am I incorrectly calling screen mode switching methods?
Big thanks!
P.S.: graphics api that i use: d3d11, opengl 4.2 - 4.6(new context).