Hi!
I have a function (see below) where float2 is a simple class holding a x and y float. I use the function to draw a circular image:
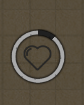
This is drawn with the variable “progress” at value 0.1 (the dark part is 10 % of a full lap). The problem is that something is off, if i evenly increase “progress” the filling of the image is faster in cardinal directions (north, east etc) and slower in the “corners” (northeast etc). The image is a square so it seems the code below goes around the other border of that square or something ( it needs to “go further” in the corners of the image). Any idea? I just want the increase to look smooth and fill the image “the same speed” no matter where in the circle im adding to the variable “progress”.
Thanks!
float2 p[4];
float rad = progress * PI2;
p[2] = float2(sin(rad), -cos(rad));
p[2].x *= sqrt(2.0) * 0.5;
p[2].y *= sqrt(2.0) * 0.5;
p[2].x += 0.5;
p[2].y += 0.5;
clamp(p[2].x, 0, 1);
clamp(p[2].y, 0, 1);
if (progress < 0.25)
{
p[0].set(0.5, 0);
p[1].set(1, 0);
p[3].set(0.5, 0.5);
}
else if (progress < 0.5) {
p[0].set(1, 0.5);
p[1].set(1, 1);
p[3].set(0.5, 0.5);
gfx.picPartRel(x + pic->GetWidth()*0.5*scale, y, pic, 0.5, 0, 0.5, 0.5, 0, scale, color, colorMode, 0, z);
}
else if (progress < 0.75) {
p[0].set(0.5, 1);
p[1].set(0, 1);
p[3].set(0.5, 0.5);
gfx.picPartRel(x + pic->GetWidth()*0.5*scale, y, pic, 0.5, 0, 0.5, 1.0, 0, scale, color, colorMode, 0, z);
}
else {
p[0].set(0, 0.5);
p[1].set(0, 0);
p[3].set(0.5, 0.5);
gfx.picPartRel(x + pic->GetWidth()*0.5*scale, y, pic, 0.5, 0, 0.5, 1.0, 0, scale, color, colorMode, 0, z);
gfx.picPartRel(x, y + pic->GetHeight()*0.5*scale, pic, 0, 0.5, 0.5, 0.5, 0, scale, color, colorMode, 0, z);
}
pictureFourPoints(x, y, p, pic, scale, color, colorMode, z);