Hello,
consider following diagram of vectors to derive the vector representing the acceleration:
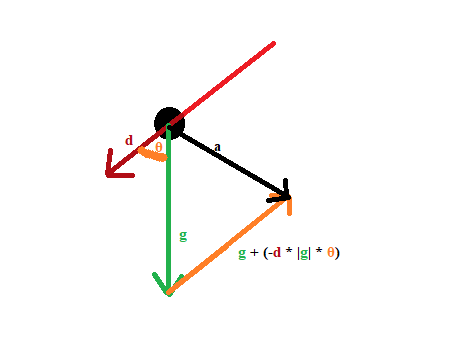
I am using the eulerian method to numerically intergrate the formula of acceleration shown in the diagram.
Here is the whole c#-class
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
Vector2 center = new Vector2(500, 250);
Vector2 bobPosition = new Vector2(450, 250);
Vector2 bobVelocity = new Vector2(0, 0);
Vector2 gravitationalForce = new Vector2(0, 10);
private void Form1_Load(object sender, EventArgs e)
{
this.Size = new Size(1000, 1000);
this.DoubleBuffered = true;
this.Paint += new PaintEventHandler(draw);
Application.Idle += new EventHandler((a, b) =>
{
if (!mousdown)
{
Vector2 dir = bobPosition - center;
dir.Normalize();
Vector2 force = new Vector2(gravitationalForce.X, gravitationalForce.Y);
force.Normalize();
float forceMagnitude = gravitationalForce.Length();
float theta = Vector2.Dot(dir, force);
dir = -dir;
Vector2 acceleration = gravitationalForce + dir * theta * (forceMagnitude);
bobVelocity += acceleration * 0.05f;
bobPosition += bobVelocity * 0.05f;
}
this.Invalidate();
});
}
private void draw( object sender, PaintEventArgs e)
{
Graphics g = e.Graphics;
g.FillEllipse(Brushes.Red, center.X - 25f, center.Y - 25f, 50, 50);
g.FillEllipse(Brushes.Blue, bobPosition.X - 12.5f, bobPosition.Y - 12.5f, 25, 25);
g.DrawLine(Pens.Black, center.X, center.Y, bobPosition.X, bobPosition.Y);
}
bool mousdown;
private void Form1_MouseDown(object sender, MouseEventArgs e)
{
mousdown = true;
}
private void Form1_MouseMove(object sender, MouseEventArgs e)
{
if (!mousdown)
return;
bobPosition = new Vector2(e.X, e.Y);
Invalidate();
}
private void Form1_MouseUp(object sender, MouseEventArgs e)
{
mousdown = false;
}
}
But the simulation is just wrong: The bob keeps falling down without ever being pushed towards center. Why is it?
Is my diagram wrong?
Best regards