I managed to produce normals in the pixel shader using the screen-space derivates of the view position from the domain shader like this
[domain("quad")]
DomainOut domain_main(PatchTess patchTess, float2 uv : SV_DomainLocation, const OutputPatch<HullOut, 4> quad)
{
DomainOut ret;
float2 topMidpointWorld = lerp( quad[ 0 ].mWorldPosition.xz, quad[ 1 ].mWorldPosition.xz, uv.x );
float2 bottomMidpointWorld = lerp( quad[ 3 ].mWorldPosition.xz, quad[ 2 ].mWorldPosition.xz, uv.x );
float2 midPointWorld = lerp( topMidpointWorld, bottomMidpointWorld, uv.y );
float3 topMidpointN = lerp( quad[ 0 ].mWorldNormal.xyz, quad[ 1 ].mWorldNormal.xyz, uv.x );
float3 bottomMidpointN = lerp( quad[ 3 ].mWorldNormal.xyz, quad[ 2 ].mWorldNormal.xyz, uv.x );
float3 midPointN = lerp( topMidpointN, bottomMidpointN, uv.y );
float2 topMidpointTexcoord = lerp( quad[ 0 ].mTexcoord, quad[ 1 ].mTexcoord, uv.x );
float2 bottomMidpointTexcoord = lerp( quad[ 3 ].mTexcoord, quad[ 2 ].mTexcoord, uv.x );
float2 midPointTexcoord = lerp( topMidpointTexcoord, bottomMidpointTexcoord, uv.y );
float y = quad[ 0 ].mWorldPosition.y;
y += ( gHeightmap.SampleLevel( gPointSampler, midPointTexcoord, 0.0f ).r * gHeightModifier );
ret.mPosition = float4( midPointWorld.x, y, midPointWorld.y, 1 );
ret.mViewPosition = mul( gFrameView, ret.mPosition );
ret.mPosition = mul( gFrameViewProj, ret.mPosition );
ret.mTexcoord = midPointTexcoord;
return ret;
}
struct PixelOut
{
float4 mDiffuse : SV_Target0;
float3 mNormal : SV_Target1;
};
PixelOut ps_main(DomainOut input)
{
PixelOut ret;
float3 dFdxPos = ddx( input.mViewPosition.xyz );
float3 dFdyPos = ddy( input.mViewPosition.xyz );
ret.mNormal = normalize( cross( dFdxPos, dFdyPos ) );
ret.mNormal += 1.0f;
ret.mNormal *= 0.5f;
ret.mDiffuse = float4( 0.0f, 1.0f, 1.0f, 1.0f);
return ret;
}
It gives me flat shading per tessellated vertex however
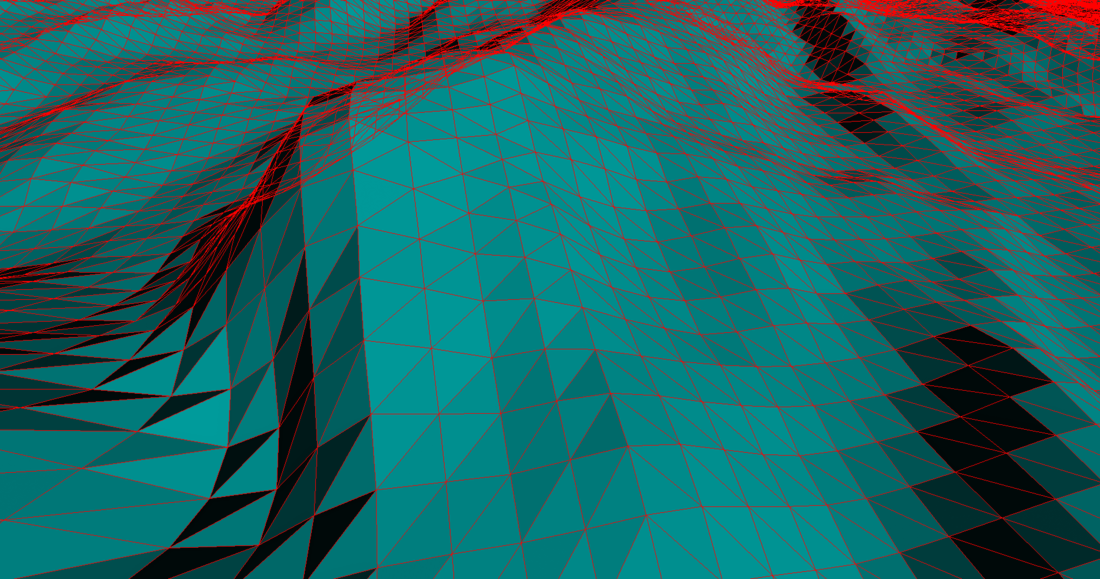
Is there a better way to produce actual smooth normals? This just isn't good enough ?