48 minutes ago, Prince Ejassa said:
Blueprints really are appealing, I can find a thing here and there on youtube and documentation but is this the right or approach? Or should I force myself to learn it the hardway (coding)?
Blueprints is coding. It's not a easier or harder way to learn, but if your a visual person it does help see things clearly.
I made this to explain it:
This is a Unity game script. It prints: 120 followed by 1 2 and 0.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class TheUnityVersion : MonoBehaviour {
int AddTwoNumbers(int NumberA, int NumberB){
return NumberA + NumberB;
}
void PrintEveryChar(string InString){
foreach (char Character in InString) {
print (Character);
}
}
// Use this for initialization
void Start () {
string TempString = AddTwoNumbers (100, 20).ToString();
print (TempString);
PrintEveryChar (TempString);
}
}
This is the blueprint version of the exact same code: prints 120 followed by 1 2 and 0.
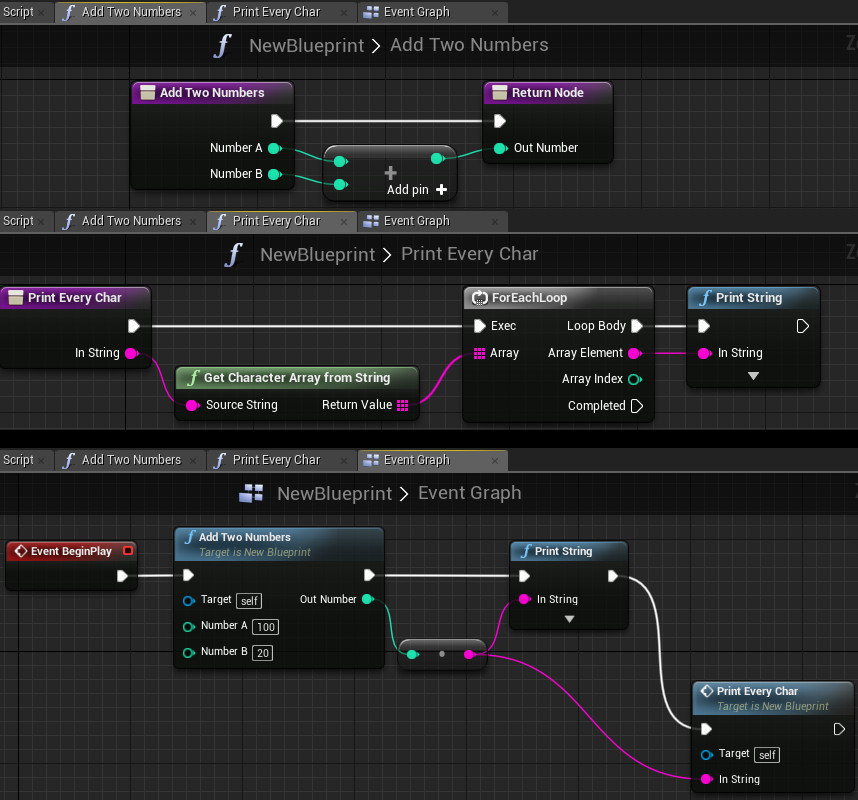
Look at the above example and decide what is easier for you to understand.
55 minutes ago, Prince Ejassa said:
Unreal engine really seems appealing but so does Game Maker Studio 2.
Unreal does have nice UI tools and 2D tools but gives you nothing special for 2D game development.
Considering how hard Unreal is to learn, the best reason to use it for 2D games is because you plan on using the Unreal engine in the long run; so you do it to learn to use Unreal.
Unity is my 2D engine of choice(it's 3D is meh), it use to be Cocos2D but knowing Unity and Unreal makes my work as a artist easier; as they are the most used engines. Game maker is also great, I started on it.
1 hour ago, Prince Ejassa said:
For the game art, I am a pretty neat drawer but that's about it, I would like to know how most of you do your game assets, do you do it by yourself or is it outsourced? or even both?
Don't worry about the art. It's easy to make a full game from start to end just using placeholder art.
Then later if your own drawing doesn't look good you can hire a artist. It's much easier for us artist to create art when we can already see from the place holders how things should work.
1 hour ago, Prince Ejassa said:
really just need someone to guide me a little bit, I have the story all played out in my head and some of it even written down, I really just need to dive in but I want to avoid feeling drowned...
Write things down as they pop into your head. Everything you write down, any idea you draw, any action you make towards the game allows it to progress.
You can try starting from the main menu and design it as players will play it; but most developers don't like working like this. Honestly there is no starting point for making games.
Making a mistake is much-much better than doing nothing. So when you don't know what to do to progress do anything.
Making games is like a never ending ocean of concepts and principles. In the end you really won't find a clear starting point and all you can do is dive in and start.