How do processors work?
I was thinking about CPUs the other day and I started wondering... How do they work? How are circuits designed that can be programmed? I can program really well, but I'm curious how it is possible. Does RAM store a charge that's sent to the processor in sequential order of on and off charges? And the processor is a circuit that does different things depending on the order? Basically, I want to know how someone could make a computer (however simple) with nothing but basic electronic components (like capacitors, diodes, wires, resistors, etc). I'm not actually trying to do this; I'm just curious how everything works.
My knowledge is not exhaustive -- pretty much limited to a digital logic class -- but:
A basic processor consists of a bunch of registers made of n-bit Flip-flops (or Latches, I think). These are apparently (read: Wikipedia) implemented in hardware like this:
http://upload.wikime...p_by_trexer.png
From there, a control circuit can take an input to determine an operation, as well as which registers to use as data input (and output) regarding the operation. These operations are hardwired as their own circuits.
It's fairly difficult to conceptualize a CPU in terms of its base electrical components. That's why they're usually modeled in terms of modules consisting of logic gates. As long as you know how to build these gates in hardware, you can break the task down. Keep in mind this is a very basic CPU I'm describing. Look into the various types of logic gates, Adders, decoders, multiplexers, latches, and flip-flops for a general outline of what's needed.
I've been trying to find a schematic for a CPU, but all of the stuff I can find is way too complicated.
A basic processor consists of a bunch of registers made of n-bit Flip-flops (or Latches, I think). These are apparently (read: Wikipedia) implemented in hardware like this:
http://upload.wikime...p_by_trexer.png
From there, a control circuit can take an input to determine an operation, as well as which registers to use as data input (and output) regarding the operation. These operations are hardwired as their own circuits.
It's fairly difficult to conceptualize a CPU in terms of its base electrical components. That's why they're usually modeled in terms of modules consisting of logic gates. As long as you know how to build these gates in hardware, you can break the task down. Keep in mind this is a very basic CPU I'm describing. Look into the various types of logic gates, Adders, decoders, multiplexers, latches, and flip-flops for a general outline of what's needed.
I've been trying to find a schematic for a CPU, but all of the stuff I can find is way too complicated.
Does RAM store a charge that's sent to the processor in sequential order of on and off charges?
*In a simple theoretical machine, an instruction may request that memory be copied from RAM to a register. The target memory address location may be placed in a register. From there the address bus is activated, then a multiplexer decodes the memory location to a specific physical block and cell of ram, and activates a path from the CPU to the that cell and to the final register. Then an "integer" ("1111 1111 ...") is sent through that path, AND'd with the contents of that cell ("1011 0011 ....") and is copied into the final register.
(*Information may or may not be correct and/or out of date, as I have only have had an introductory course in computer architecture.)
1. How do they work?
2. How are circuits designed that can be programmed? I can program really well, but I'm curious how it is possible.
3. Does RAM store a charge that's sent to the processor in sequential order of on and off charges?
4. And the processor is a circuit that does different things depending on the order?
5. Basically, I want to know how someone could make a computer (however simple) with nothing but basic electronic components (like capacitors, diodes, wires, resistors, etc). I'm not actually trying to do this; I'm just curious how everything works.
Google is your friend.
1. This could be answered many ways. They work using semiconductors that are traced out in patterns on a chip that work as diodes, transistors, etc., to have a controlled flow of energy. These are combined in common patterns such as "logic gates", which are combined into larger and more creative patterns, which are combined into even more patterns, repeated many many times, until you have a modern CPU. You can study this to a PhD level and beyond in terms of physics, or in terms of chemistry, or in terms of electrical engineering and computer engineering. Google shows lots of links ranging from really stupid sites up through post-graduate technical papers.
2. Study computer theory. The theory behind it gets complicated very quickly. Basically it is an implementation of a Turing Machine, which is the fundamentally basic theoretical computer. (I love that site. It is very visual and explains the concepts neatly.) One amazing feature of a Turing Machine is that you can have a program as data. The biggest point of a Turing Machine is that the data is the computer. That's all a CPU core is: a program running a program running a program that is all etched on a semiconductor die. It is hardware that has hard-coded software written on it, which runs another piece of hard-coded software, and then you call that second piece of software the CPU. That in turn runs your program as data. The first machine is the actual hardware that is burnt into metal. The second machine is a program that runs on the first machine, which knows how to interpret the bytes of data that your program is composed of. Then you get an operating system, which adds another layer of programs on top of that. Repeat many times.
3. Study electrical engineering. Almost all the components in your computers are microcontrollers. That includes memory chips. There are many hobby microcontrollers that you can build yourself, if that is your interest. Just read the schematics (if you are able) to get a feel for how they work. Generally they require a reasonable background in electronics to make them. The common DRAM chip in your computer is basicallya tiny microcontroller on the front end plastered to a bank of several hundred billion of capacitors on the back end.
4. Yes and no. See the answer 2 above. It is a software program running on a hardware program running on another hardware program running on powered-up processed silicon.
5. Yes, see the link in answer 3 above.
1) Express your operation in boolean algebra, for exemple the addition of 2 bits gives
A B Sum Carry
0 0 0 0
0 1 1 0
1 0 1 0
1 1 0 1
which gives :
Sum = A XOR B
Carry = A AND B
For simplicity of design, express these equations to use a single logic gate, for exemple a NAND gate
Sum = ( A NAND ( A NAND B ) ) NAND ( B NAND ( A NAND B ) )
Carry = ( A NAND B) NAND ( A NAND B )
2) take a few resistors and transistors to make the NAND gates :
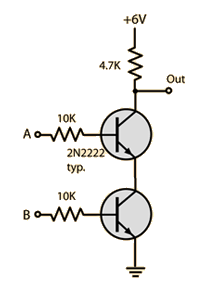
3) Wire the gates according to the equation, and you have a mighty processor able to add 2 numbers of 1 bit.
4) Combine several of this to add bigger numbers, 8 of them allows to add 2 8-bits numbers.
5) Do the same for each operation you want to support.
Of course you have a lot more going on in a real processor (memorizing state, operation selection, address decoding, errors flags...) but the basics is here and everything can be express as NAND gates wich is just 2 transistors.
A B Sum Carry
0 0 0 0
0 1 1 0
1 0 1 0
1 1 0 1
which gives :
Sum = A XOR B
Carry = A AND B
For simplicity of design, express these equations to use a single logic gate, for exemple a NAND gate
Sum = ( A NAND ( A NAND B ) ) NAND ( B NAND ( A NAND B ) )
Carry = ( A NAND B) NAND ( A NAND B )
2) take a few resistors and transistors to make the NAND gates :
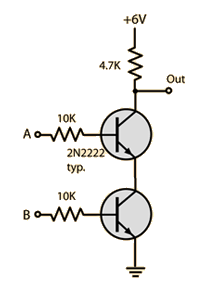
3) Wire the gates according to the equation, and you have a mighty processor able to add 2 numbers of 1 bit.
4) Combine several of this to add bigger numbers, 8 of them allows to add 2 8-bits numbers.
5) Do the same for each operation you want to support.
Of course you have a lot more going on in a real processor (memorizing state, operation selection, address decoding, errors flags...) but the basics is here and everything can be express as NAND gates wich is just 2 transistors.
If you really want to learn these things, a good way would be to pick up an experiment kit. You don't see these about so much any more, but they were the way that a lot of people my age learned about electronics.
As examples; http://www.cheekytronics.com/Projects/electronic_construction_kits.html -- Amazon apparently sells them.
Actually building your own computer is perfectly possible -- although I'd recommend starting with something a bit higher than transistors. It's entirely reasonable to breadboard 8-bit CPUs, static RAM. Burn software into EPROMs and build keyboards and 7-segment displays. None of this costs a huge amount, and the experience of watching the things actually running (via a borrowed oscilloscope!) is a brilliant basis for understanding more complex systems.
As examples; http://www.cheekytronics.com/Projects/electronic_construction_kits.html -- Amazon apparently sells them.
Actually building your own computer is perfectly possible -- although I'd recommend starting with something a bit higher than transistors. It's entirely reasonable to breadboard 8-bit CPUs, static RAM. Burn software into EPROMs and build keyboards and 7-segment displays. None of this costs a huge amount, and the experience of watching the things actually running (via a borrowed oscilloscope!) is a brilliant basis for understanding more complex systems.
Ive had one full year worth of architecture classes teaching this stuff. I don't think it will be thoroughly explainable in a forum post. There are some good books on the subject though, its cool stuff,
Never, ever stop learning.
- Me
- Me
A few years back I bought a package from xgamestation.com and was pretty pleased with it. The one that I ordered came with a few books (some were on a disk) which seemed to cover just about everything that I took in a 2 year computer engineering course several years before. In the end it's slanted towards game development but the electronics and microcontroller stuff would be relevant for whatever project you wanted.
Ive had one full year worth of architecture classes teaching this stuff. I don't think it will be thoroughly explainable in a forum post. There are some good books on the subject though, its cool stuff,
Indeed. Even with my undergraduate and graduate architecture classes I can say with confidence that it's not going to be explained here. Speaking of books though there is a nice Computer Architecture book. I'll grab a link to it when I get home. I can't remember the full title.
// Edit here is the link.
Some advanced reading material which kind of assumes a basic understanding of digital electronics and basic computer architecture. Covers fun things like vector pipelining and the theory and implementation of it in respect to functional units (core operations in a processor).
This topic is closed to new replies.
Advertisement
Popular Topics
Advertisement
Recommended Tutorials
Advertisement