I thought I had smoothly working transparency, until I noticed some objects were being drawn on top of transparent objects which they should have been behind of. When I started playing with glDepthMask I was unable to find a simple solution. Here is an illustration:
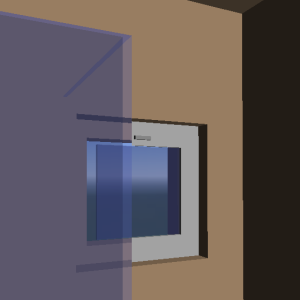
With glDepthMask being disabled for drawing the transparent objects, then enabled for the opaque. Btw, that's a window behind a transparent shower cabinet - see how the window frame, which is a separate object, disappears completely behind the shower glass.
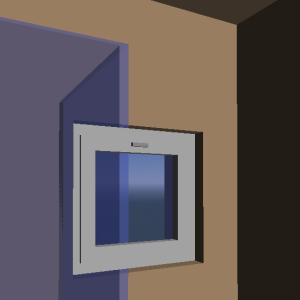
With glDepthMask not being called at all when drawing transparent object (it is on by default). The window frame is now drawn above the shower glass (?)
And here is the rendering code:
void RenderScene ( Mscene *s )
{
unsigned int a, b, c;
for ( a = 0; a < s->numObj; a++ )
{
for ( b = 0; b < s->obj[a].numMesh; b++ )
{
glColor4ub( s->obj[a].mesh[b].col[0], s->obj[a].mesh[b].col[1], s->obj[a].mesh[b].col[2], s->obj[a].mesh[b].opac );
// If mesh is transparent, enable transparency
if ( s->obj[a].mesh[b].opac < 255 )
{
glEnable(GL_BLEND);
glDepthMask(GL_FALSE);
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
}
if ( s->obj[a].mesh[b].textured )
glBindTexture ( GL_TEXTURE_2D, s->obj[a].mesh[b].texID );
glBegin(GL_TRIANGLES);
for ( MVEuint16 c = 0; c < s->obj[a].mesh[b].numTri; c++ )
{
glTexCoord2fv( s->obj[a].tc[s->obj[a].mesh[b].tri[c].ti[0]] );
glVertex3fv( s->obj[a].vert[s->obj[a].mesh[b].tri[c].vi[0]] );
glTexCoord2fv( s->obj[a].tc[s->obj[a].mesh[b].tri[c].ti[1]] );
glVertex3fv( s->obj[a].vert[s->obj[a].mesh[b].tri[c].vi[1]] );
glTexCoord2fv( s->obj[a].tc[s->obj[a].mesh[b].tri[c].ti[2]] );
glVertex3fv( s->obj[a].vert[s->obj[a].mesh[b].tri[c].vi[2]] );
}
glEnd();
// If mesh is transparent, disable transparency
if ( s->obj[a].mesh[b].opac < 255 )
{
glDepthMask(GL_TRUE);
glDisable(GL_BLEND);
}
}
}
}
Can anyone explain the logic behind drawing multiple transparent and opaque objects, I find the OpenGL doc is a bit mystical on these issues.
[edited by - Keermalec on October 8, 2003 5:21:15 PM]