After looking and asking about, I made an assembla page for Tangent. Included is a release for version 0.1 and some wiki/documentation stuff. It should only require .NET 2.0, and ideally just extract from zip and run the exe.
Previous Entry
Optimization Tidbit
Next Entry
Tangent: Inheritance Bugs
Comments
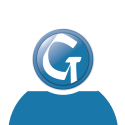
Daerax
August 03, 2008 01:47 AM
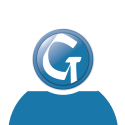
Now that Ive been playing with your code it looks like what you are doing is kinda like implementation inheritance via intersection of types (this is related too but not quite the same as the notion of intersection types since this relates strictly to inheritance).
So like suppose HasName = {string} and pirate = {string, int, int}
then HasName s = new pirate seems to be like tyepof s = typeof HasName ∩ typeof pirate = {string} where HasName ⊂ Pirate. So given abstract a, and concrete type t. then binding o : a to t() results in o : a where x in a => x in t . So the abstraction seems to be a restriction on Pirate. Ideally it should ;create an object that is a subset of t or can stand in for type t but this does not seem to work
I saw the section on inheritance and mixins but cant seem to find it anymore. Want to play with that some.
Will leave the below as an example of what not to do hehe.
So like suppose HasName = {string} and pirate = {string, int, int}
then HasName s = new pirate seems to be like tyepof s = typeof HasName ∩ typeof pirate = {string} where HasName ⊂ Pirate. So given abstract a, and concrete type t. then binding o : a to t() results in o : a where x in a => x in t . So the abstraction seems to be a restriction on Pirate. Ideally it should ;create an object that is a subset of t or can stand in for type t but this does not seem to work
I saw the section on inheritance and mixins but cant seem to find it anymore. Want to play with that some.
Will leave the below as an example of what not to do hehe.
public class HasNameAge{
public abstract string Name;
public abstract int Age ;
}
public class Pirate{
public int Age = 20;
public int Hp = 5;
public string Name = "BlackBeard";
}
public static void PrintAge(Pirate hna) // Failed cause Pirate not <: HasNamAge
{
print (hna.Age);
}
HasNameAge Pete = new Pirate;
public static void main(){
print "Hello World ";
print Pete.Name;
//print Pete.Hp;
PrintAge(Pete);
}
August 03, 2008 10:22 AM
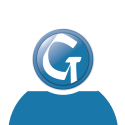
Enh... not so much. When you have HasName x = new Pirate;, the variable x is of type HasName. That's all the compiler knows, and cares about. It's a guarantee that the object will have a Name, so that's all you can use. The object is still a Pirate (so you can cast it back to Pirate once casting works), but the compiler checks that Pirate satisfied the guarantee that the object has a Name. No actual intersection there. And it will at this point give you errors if you try to assign something that is not a sub-type to a variable of a type.
An aside: Though intersection is in the code, and is used. It's currently only used when determining types for type inference during a generic method invocation. If a method with signature void(T,T) is passed an object,string it will properly infer that T is object. It also (perhaps unintuitively) if passed (AB,BC) will intersect the two, and allow you to call members of B. I am unsure if I'll provide intersection as a user callable Type expression operator yet. It seems to have limited uses, a little awkwardness, and tends to be unintuitive.
And no, you can't declare generic methods yet, but a little of the under-the-hood stuff is. The assignment operator's type for example is defined as operator void<T> assign(T,U) where U:T.
An aside: Though intersection is in the code, and is used. It's currently only used when determining types for type inference during a generic method invocation. If a method with signature void(T,T) is passed an object,string it will properly infer that T is object. It also (perhaps unintuitively) if passed (AB,BC) will intersect the two, and allow you to call members of B. I am unsure if I'll provide intersection as a user callable Type expression operator yet. It seems to have limited uses, a little awkwardness, and tends to be unintuitive.
And no, you can't declare generic methods yet, but a little of the under-the-hood stuff is. The assignment operator's type for example is defined as operator void<T> assign(T,U) where U:T.
August 03, 2008 10:47 AM
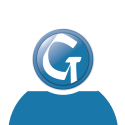
Agreed intersection types are unweildy, especially on .NET I do not think it would really be worth it.
I was editing while you replied so my last post was basically nonsense. Hopefully the edited one makes more sense. Its just me trying to understand the language. In terms of intersection I meant basic set intersection, was just trying to express an abstraction of the code.
This is quite flexible way to program with interface. Cool!
Well that is my report. =)
I was editing while you replied so my last post was basically nonsense. Hopefully the edited one makes more sense. Its just me trying to understand the language. In terms of intersection I meant basic set intersection, was just trying to express an abstraction of the code.
This is quite flexible way to program with interface. Cool!
public static void PrintAge(HasNameAge hna)
{
local HasNameAge h = hna;
print (h.Age);
}
Pirate Pete = new Pirate;
public static void main(){
print "Hello World ";
print Pete.Name;
//print Pete.Hp;
PrintAge(Pete);
Well that is my report. =)
August 03, 2008 11:30 AM
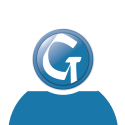
Quote:
I saw the section on inheritance and mixins but cant seem to find it anymore. Want to play with that some.
maybe?
Type Declarations
Implications of types as sets #2
If I remember correctly, declaring an inheritor in the type definition is not guaranteed to work in v0.1; I don't remember what in particular doesn't work. Probably anything involving name collisions, maybe inheriting goose types... not sure. [edit: hrm, looks like even the simple stuff isn't there yet. Oh well, it's in the queue.]
What should happen is that all members of the inheritee are mixed with the members declared in the body, yielding a new type which is the union of the two.
August 03, 2008 02:11 PM
Advertisement
Latest Entries
And so it goes...
1787 views
Tangent, now with usable error messages!
1929 views
Back to work!
1859 views
Tangent: Haitus
1807 views
Tangent: Syntax reworking
1593 views
Tangent: Adverbs
1456 views
Tangent: Pattern Matching, Part 1
1668 views
Tangent: Pattern Matching, Part 1
1520 views
Tangent: Generic Method Bodies, Part 2
1681 views
Tangent: Generic Method Bodies, Part 1
1528 views
Advertisement